用C语言写一段代码:oop租车系统(多重继承)
时间: 2024-01-13 15:02:57 浏览: 78
抱歉,C语言不支持多重继承,因为它不是一种面向对象的语言。不过,你可以使用结构体来模拟类和对象的概念。下面是一个简单的示例代码,展示了如何使用结构体来实现一个租车系统:
```
#include <stdio.h>
#include <string.h>
// 定义车辆结构体
struct Vehicle {
char make[20]; // 品牌
char model[20]; // 型号
int year; // 年份
int rent_per_day; // 租金/天
};
// 定义客户结构体
struct Customer {
char name[30]; // 姓名
int age; // 年龄
struct Vehicle *rented_vehicle; // 租用的车辆
};
// 定义租车公司结构体
struct CarRentalCompany {
struct Vehicle *vehicles; // 所有车辆的数组
int num_vehicles; // 车辆数量
struct Customer *customers; // 所有客户的数组
int num_customers; // 客户数量
};
// 初始化车辆
void init_vehicles(struct Vehicle *vehicles, int num_vehicles) {
for (int i = 0; i < num_vehicles; i++) {
sprintf(vehicles[i].make, "Make%d", i+1);
sprintf(vehicles[i].model, "Model%d", i+1);
vehicles[i].year = 2020;
vehicles[i].rent_per_day = 50 + i*10;
}
}
// 初始化客户
void init_customers(struct Customer *customers, int num_customers) {
for (int i = 0; i < num_customers; i++) {
sprintf(customers[i].name, "Customer%d", i+1);
customers[i].age = 20 + i*5;
customers[i].rented_vehicle = NULL;
}
}
// 打印车辆信息
void print_vehicle(const struct Vehicle *vehicle) {
printf("%s %s (%d) - $%d/day\n",
vehicle->make, vehicle->model, vehicle->year, vehicle->rent_per_day);
}
// 打印客户信息
void print_customer(const struct Customer *customer) {
printf("%s (%d years old)\n", customer->name, customer->age);
if (customer->rented_vehicle != NULL) {
printf("Rented vehicle: ");
print_vehicle(customer->rented_vehicle);
}
}
// 打印所有车辆信息
void print_all_vehicles(const struct Vehicle *vehicles, int num_vehicles) {
printf("All vehicles:\n");
for (int i = 0; i < num_vehicles; i++) {
print_vehicle(&vehicles[i]);
}
}
// 打印所有客户信息
void print_all_customers(const struct Customer *customers, int num_customers) {
printf("All customers:\n");
for (int i = 0; i < num_customers; i++) {
print_customer(&customers[i]);
}
}
// 租用车辆
void rent_vehicle(struct Customer *customer, struct Vehicle *vehicle) {
customer->rented_vehicle = vehicle;
printf("%s rented ", customer->name);
print_vehicle(vehicle);
}
int main() {
// 初始化租车公司
struct Vehicle vehicles[3];
init_vehicles(vehicles, 3);
struct Customer customers[2];
init_customers(customers, 2);
struct CarRentalCompany company = {vehicles, 3, customers, 2};
// 打印所有车辆和客户信息
print_all_vehicles(company.vehicles, company.num_vehicles);
print_all_customers(company.customers, company.num_customers);
// 客户1租用第2辆车
rent_vehicle(&company.customers[0], &company.vehicles[1]);
print_customer(&company.customers[0]);
return 0;
}
```
该代码定义了三个结构体:Vehicle、Customer 和 CarRentalCompany。它们分别表示车辆、客户和租车公司。其中,CarRentalCompany 包含了所有车辆和客户的信息。
使用该代码,我们可以创建一个租车公司,并初始化其中的车辆和客户。然后,我们可以打印所有车辆和客户的信息,以及让某个客户租用某辆车。
阅读全文
相关推荐
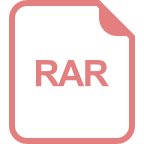
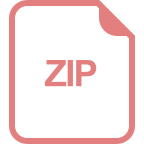
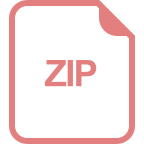
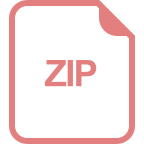
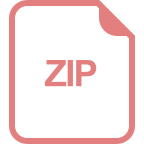
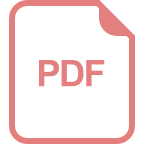
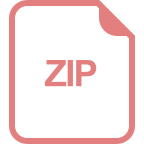
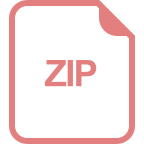
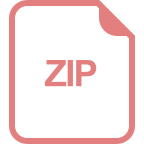
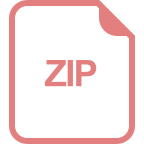
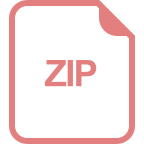
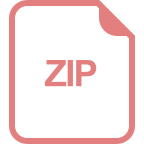
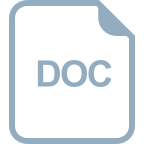
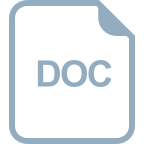
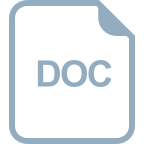
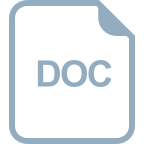