android获取GPU使用率
时间: 2023-10-18 13:05:19 浏览: 45
要获取 Android 设备的 GPU 使用率,你可以使用 Android Debug Bridge (ADB) 命令行工具。首先,你需要在电脑上安装 ADB。然后,在命令行窗口中输入以下命令:
```
adb shell dumpsys gfxinfo
```
这将会显示有关设备 GPU 的信息,包括 GPU 进程使用情况、渲染帧数以及帧速率。要查看更多信息,你可以使用以下命令:
```
adb shell dumpsys gfxinfo <package_name>
```
其中 `<package_name>` 是你想要查看的应用的包名。
如果你想在应用内查看 GPU 使用率,你可以使用 Android Profiler 工具,它可以在 Android Studio 中找到。在 Android Profiler 中,你可以选择“GPU”选项卡来查看 GPU 使用情况。
此外,你也可以使用第三方应用来查看 GPU 使用情况,比如 GPU-Z 和 AIDA64。
相关问题
android 获取gpu内存使用率
要获取Android设备上GPU内存使用率,可以使用以下步骤:
1. 在Android Studio中打开您的应用程序项目。
2. 在项目中添加以下依赖项:
```xml
dependencies {
implementation 'com.android.support:appcompat-v7:28.0.0'
implementation 'com.android.support:support-annotations:28.0.0'
implementation 'com.android.support:design:28.0.0'
implementation 'com.android.support:support-v4:28.0.0'
implementation 'com.android.support:cardview-v7:28.0.0'
implementation 'com.android.support:recyclerview-v7:28.0.0'
implementation 'com.android.support:multidex:1.0.3'
implementation 'com.google.android.gms:play-services-ads:17.2.0'
implementation 'com.google.android.gms:play-services-vision:17.0.2'
implementation 'com.google.android.gms:play-services-location:16.0.0'
implementation 'com.google.android.gms:play-services-maps:16.0.0'
implementation 'com.google.android.gms:play-services-auth:16.0.1'
implementation 'com.google.android.gms:play-services-identity:16.0.0'
implementation 'com.google.android.gms:play-services-games:16.0.0'
implementation 'com.google.android.gms:play-services-drive:16.0.0'
implementation 'com.google.android.gms:play-services-plus:16.0.0'
implementation 'com.google.android.gms:play-services-panorama:16.0.0'
implementation 'com.google.android.gms:play-services-nearby:16.0.0'
}
```
3. 在应用程序中添加以下代码:
```java
private double getGpuUsage() {
Process process = null;
double gpuUsage = 0.0d;
try {
process = Runtime.getRuntime().exec("dumpsys meminfo");
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()), 1024);
String line;
int index = -1;
while ((line = reader.readLine()) != null) {
if (line.contains("Graphics")) {
index = line.indexOf(":");
gpuUsage = Double.parseDouble(line.substring(index + 1, line.length() - 1).trim());
break;
}
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (process != null) {
process.destroy();
}
}
return gpuUsage;
}
```
4. 调用此方法以获取GPU内存使用率:
```java
double gpuUsage = getGpuUsage();
```
5. 您可以将此值打印到控制台中,也可以将其发送到您的服务器以进行分析和监控。
android 获取gpu 内存使用率
可以通过使用Android系统提供的OpenGL ES API来获取GPU内存使用率。
1. 首先,在AndroidManifest.xml文件中添加以下权限:
```xml
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.GET_TASKS"/>
<uses-permission android:name="android.permission.REAL_GET_TASKS"/>
```
2. 在代码中,可以使用如下方式获取OpenGL ES的状态信息:
```java
import android.app.ActivityManager;
import android.content.Context;
import android.opengl.GLSurfaceView;
import javax.microedition.khronos.opengles.GL10;
public class OpenGLMonitor implements GLSurfaceView.Renderer {
private ActivityManager mActivityManager;
private int mMaxTextureSize;
private int mMaxTextureUnits;
private int mMaxVertexAttribs;
private int mMaxVaryingVectors;
private int mMaxVertexUniformComponents;
private int mMaxFragmentUniformComponents;
private int mMaxVertexTextureImageUnits;
private int mMaxFragmentTextureImageUnits;
private int mMaxRenderbufferSize;
private int mMaxViewportDims;
public OpenGLMonitor(Context context) {
mActivityManager = (ActivityManager)context.getSystemService(Context.ACTIVITY_SERVICE);
}
@Override
public void onSurfaceCreated(GL10 gl, javax.microedition.khronos.egl.EGLConfig config) {
mMaxTextureSize = getMaxTextureSize(gl);
mMaxTextureUnits = getMaxTextureUnits(gl);
mMaxVertexAttribs = getMaxVertexAttribs(gl);
mMaxVaryingVectors = getMaxVaryingVectors(gl);
mMaxVertexUniformComponents = getMaxVertexUniformComponents(gl);
mMaxFragmentUniformComponents = getMaxFragmentUniformComponents(gl);
mMaxVertexTextureImageUnits = getMaxVertexTextureImageUnits(gl);
mMaxFragmentTextureImageUnits = getMaxFragmentTextureImageUnits(gl);
mMaxRenderbufferSize = getMaxRenderbufferSize(gl);
mMaxViewportDims = getMaxViewportDims(gl);
}
@Override
public void onSurfaceChanged(GL10 gl, int width, int height) {
}
@Override
public void onDrawFrame(GL10 gl) {
}
private int getMaxTextureSize(GL10 gl) {
int[] params = new int[1];
gl.glGetIntegerv(GL10.GL_MAX_TEXTURE_SIZE, params, 0);
return params[0];
}
private int getMaxTextureUnits(GL10 gl) {
int[] params = new int[1];
gl.glGetIntegerv(GL10.GL_MAX_TEXTURE_UNITS, params, 0);
return params[0];
}
private int getMaxVertexAttribs(GL10 gl) {
int[] params = new int[1];
gl.glGetIntegerv(GL10.GL_MAX_VERTEX_ATTRIBS, params, 0);
return params[0];
}
private int getMaxVaryingVectors(GL10 gl) {
int[] params = new int[1];
gl.glGetIntegerv(GL10.GL_MAX_VARYING_VECTORS, params, 0);
return params[0];
}
private int getMaxVertexUniformComponents(GL10 gl) {
int[] params = new int[1];
gl.glGetIntegerv(GL10.GL_MAX_VERTEX_UNIFORM_COMPONENTS, params, 0);
return params[0];
}
private int getMaxFragmentUniformComponents(GL10 gl) {
int[] params = new int[1];
gl.glGetIntegerv(GL10.GL_MAX_FRAGMENT_UNIFORM_COMPONENTS, params, 0);
return params[0];
}
private int getMaxVertexTextureImageUnits(GL10 gl) {
int[] params = new int[1];
gl.glGetIntegerv(GL10.GL_MAX_VERTEX_TEXTURE_IMAGE_UNITS, params, 0);
return params[0];
}
private int getMaxFragmentTextureImageUnits(GL10 gl) {
int[] params = new int[1];
gl.glGetIntegerv(GL10.GL_MAX_TEXTURE_IMAGE_UNITS, params, 0);
return params[0];
}
private int getMaxRenderbufferSize(GL10 gl) {
int[] params = new int[1];
gl.glGetIntegerv(GL10.GL_MAX_RENDERBUFFER_SIZE, params, 0);
return params[0];
}
private int getMaxViewportDims(GL10 gl) {
int[] params = new int[2];
gl.glGetIntegerv(GL10.GL_MAX_VIEWPORT_DIMS, params, 0);
return params[0] * params[1];
}
public float getGpuUsage() {
ActivityManager.MemoryInfo memoryInfo = new ActivityManager.MemoryInfo();
mActivityManager.getMemoryInfo(memoryInfo);
long totalMemory = memoryInfo.totalMem;
long availMemory = memoryInfo.availMem;
long usedMemory = totalMemory - availMemory;
float usage = (float) usedMemory / totalMemory;
return usage;
}
}
```
3. 然后,可以在需要获取GPU内存使用率的地方调用getGpuUsage()方法来获取GPU内存使用率。
```java
OpenGLMonitor monitor = new OpenGLMonitor(context);
float gpuUsage = monitor.getGpuUsage();
```
相关推荐
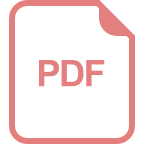












