DS18B20温度传感器数码管显示温度和报警
时间: 2024-02-14 14:06:20 浏览: 110
好的,这是一个关于温度传感器和数码管的问题。我可以给您一些思路和建议。
首先,您需要连接DS18B20温度传感器和数码管到您的控制器(比如Arduino、Raspberry Pi等)。您可以使用GPIO口或者其他接口来连接它们。
然后,您需要编写代码来读取传感器的温度值,并将其显示在数码管上。您可以使用数字转换库和数码管库来实现这个功能。同时,您还需要设置报警阈值,并在温度超过阈值时触发报警。
以下是一个简单的示例代码,用于读取DS18B20传感器的温度并将其显示在数码管上:
```C++
#include <OneWire.h>
#include <DallasTemperature.h>
#include <TM1637Display.h>
// Data wire is plugged into pin D2 on the Arduino
#define ONE_WIRE_BUS 2
// Setup a oneWire instance to communicate with any OneWire devices
OneWire oneWire(ONE_WIRE_BUS);
// Pass our oneWire reference to Dallas Temperature.
DallasTemperature sensors(&oneWire);
// Define the TM1637 display pins
#define CLK 3
#define DIO 4
// Create a TM1637Display object
TM1637Display display(CLK, DIO);
// Set the alarm threshold
const float THRESHOLD = 25.0;
void setup() {
// Start the serial communication
Serial.begin(9600);
// Start the Dallas Temperature sensor
sensors.begin();
// Set the brightness of the display
display.setBrightness(0x0f);
}
void loop() {
// Request temperature readings
sensors.requestTemperatures();
// Get the temperature value
float temperature = sensors.getTempCByIndex(0);
// Display the temperature on the TM1637 display
int temp_int = (int) temperature;
int temp_decimal = (int) ((temperature - temp_int) * 10);
int temp_digits[4] = {temp_int / 10, temp_int % 10, temp_decimal / 10, temp_decimal % 10};
display.setSegments(temp_digits);
// Check if the temperature is above the threshold
if (temperature > THRESHOLD) {
// Trigger the alarm
Serial.println("Temperature above threshold!");
}
// Delay for a short period of time
delay(1000);
}
```
这个示例代码使用了OneWire库和DallasTemperature库来读取DS18B20传感器的温度值,并使用TM1637Display库来控制数码管显示温度。同时,它还设置了一个温度阈值,如果温度超过这个阈值,就会触发一个简单的报警。
请注意,这只是一个简单的示例代码,您需要根据您的具体硬件和需求进行调整和修改。希望这个示例能够帮助您开始编写代码!
阅读全文
相关推荐









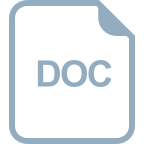








