python sqlite 判断表是否存在
时间: 2023-09-29 21:03:06 浏览: 171
在 Python 中,可以使用 sqlite3 模块连接 SQLite 数据库并执行 SQL 语句。要判断表是否存在,可以使用如下代码:
```python
import sqlite3
# 连接 SQLite 数据库
conn = sqlite3.connect('database.db')
# 创建游标对象
cursor = conn.cursor()
# 执行 SQL 语句查询表是否存在
table_name = 'my_table'
cursor.execute("SELECT name FROM sqlite_master WHERE type='table' AND name=?", (table_name,))
result = cursor.fetchone()
if result:
print('表已存在')
else:
print('表不存在')
# 关闭游标和连接
cursor.close()
conn.close()
```
其中,`sqlite_master` 是 SQLite 中的系统表,用于存储数据库的元数据信息。通过查询该表可以获取到数据库中所有的表、视图、索引等信息。上述代码中,使用了 `SELECT` 语句查询 `sqlite_master` 表中是否存在指定名称的表,如果查询结果非空,则说明该表已经存在。
相关问题
python 判断sqlite表是否存在
可以使用以下代码来判断 SQLite 数据库中的表是否存在:
```python
import sqlite3
# 连接到 SQLite 数据库
conn = sqlite3.connect('example.db')
# 获取游标
cursor = conn.cursor()
# 判断表是否存在
cursor.execute("SELECT name FROM sqlite_master WHERE type='table' AND name='table_name'")
table_exists = cursor.fetchall()
if table_exists:
print('表已经存在')
else:
print('表不存在')
# 关闭连接
conn.close()
```
请将 `example.db` 替换为你的数据库名称,将 `table_name` 替换为你要查询的表名。如果 `table_exists` 列表不为空,则表示表已经存在。
python sqlite3 判断数据表是否存在
在 Python 中,可以通过 sqlite3 模块来操作 SQLite 数据库。要判断数据表是否存在,可以使用以下代码:
```python
import sqlite3
# 连接数据库
conn = sqlite3.connect('example.db')
# 获取游标
cursor = conn.cursor()
# 判断数据表是否存在
table_name = 'my_table'
cursor.execute("SELECT name FROM sqlite_master WHERE type='table' AND name=?", (table_name,))
result = cursor.fetchone()
if result:
print(f"数据表 {table_name} 存在")
else:
print(f"数据表 {table_name} 不存在")
# 关闭游标和连接
cursor.close()
conn.close()
```
其中,`SELECT name FROM sqlite_master WHERE type='table' AND name=?` 是查询数据表的 SQL 语句,`name=?` 是 SQL 语句中的占位符,用于传入数据表名称。`cursor.execute()` 方法执行 SQL 语句,`cursor.fetchone()` 方法获取查询结果的第一条记录。如果查询结果不为空,则说明数据表存在;否则,数据表不存在。
阅读全文
相关推荐
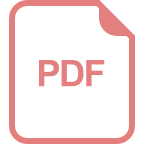
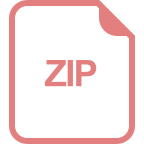
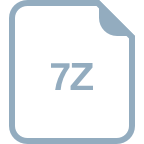













