pyqt主界面中显示子界面,等待子界面的enter按下时,子界面再close
时间: 2023-07-16 19:14:48 浏览: 88
你可以使用`QDialog`来创建子界面,然后在主界面中调用子界面的`exec_()`方法来显示它。在子界面中,你可以重写`keyPressEvent`方法来检测用户是否按下了Enter键。如果是,你可以调用`accept()`方法来关闭子界面。以下是一个简单的示例代码:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QDialog, QPushButton, QVBoxLayout, QLabel
from PyQt5.QtGui import QKeyEvent
class ChildDialog(QDialog):
def __init__(self, parent=None):
super().__init__(parent)
# 创建子界面上的控件
self.label = QLabel('Press Enter to close the dialog')
layout = QVBoxLayout()
layout.addWidget(self.label)
self.setLayout(layout)
def keyPressEvent(self, event: QKeyEvent):
if event.key() == 16777220: # Enter键的keyCode
self.accept()
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建主界面上的控件
button = QPushButton('Show dialog', self)
button.clicked.connect(self.show_dialog)
layout = QVBoxLayout()
layout.addWidget(button)
central_widget = QWidget()
central_widget.setLayout(layout)
self.setCentralWidget(central_widget)
def show_dialog(self):
# 创建子界面并显示
dialog = ChildDialog(self)
dialog.exec_()
if __name__ == '__main__':
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
```
在这个示例代码中,我们首先创建了一个`ChildDialog`类来表示子界面。在这个类中,我们创建了一个`QLabel`控件来提示用户按下Enter键来关闭子界面。我们还重写了`keyPressEvent`方法来检测用户是否按下了Enter键,如果是,我们就调用`accept()`方法来关闭子界面。
在`MainWindow`类中,我们创建了一个`QPushButton`控件来触发显示子界面的操作。当用户点击按钮时,我们创建一个`ChildDialog`对象并调用它的`exec_()`方法来显示它。因为`exec_()`方法会阻塞主线程,直到子界面被关闭,所以我们不需要显式地等待子界面被关闭。当用户按下Enter键时,子界面会自动关闭,主线程继续执行。
阅读全文
相关推荐
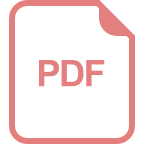
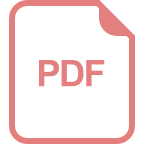
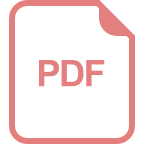















