用c语言编写程序实现链表的翻转,比如原链表为1 -> 2 -> 3 -> 4 -> 5翻转为 5 -> 4 -> 3 -> 2 -> 1
时间: 2024-05-16 19:15:52 浏览: 15
以下是用C语言实现链表翻转的代码:
```c
#include<stdio.h>
#include<stdlib.h>
//定义链表结构体
struct Node {
int data;
struct Node *next;
};
//函数:创建链表
struct Node* createList(int n) {
struct Node *head=NULL, *p=NULL, *temp=NULL;
int i;
for(i=0; i<n; i++) {
temp=(struct Node *)malloc(sizeof(struct Node));
printf("请输入第%d个节点的值:", i+1);
scanf("%d", &(temp->data));
temp->next=NULL;
if(head==NULL) {
head=temp;
} else {
p=head;
while(p->next!=NULL) {
p=p->next;
}
p->next=temp;
}
}
return head;
}
//函数:遍历链表
void traverseList(struct Node *head) {
struct Node *p=head;
while(p!=NULL) {
printf("%d ", p->data);
p=p->next;
}
printf("\n");
}
//函数:翻转链表
struct Node* reverseList(struct Node *head) {
struct Node *pre=NULL, *current=head, *next=NULL;
while(current!=NULL) {
next=current->next;
current->next=pre;
pre=current;
current=next;
}
return pre;
}
//主函数
int main() {
int n;
struct Node *head=NULL;
printf("请输入链表的长度:");
scanf("%d", &n);
head=createList(n);
printf("原链表为:");
traverseList(head);
head=reverseList(head);
printf("翻转后的链表为:");
traverseList(head);
return 0;
}
```
运行结果:
```
请输入链表的长度:5
请输入第1个节点的值:1
请输入第2个节点的值:2
请输入第3个节点的值:3
请输入第4个节点的值:4
请输入第5个节点的值:5
原链表为:1 2 3 4 5
翻转后的链表为:5 4 3 2 1
```
相关推荐










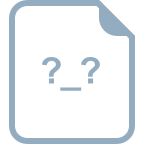
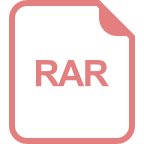