python代码,定期给不同邮箱发送不同名称邮件
时间: 2024-02-03 22:04:37 浏览: 21
以下是一个Python代码示例,用于定期发送不同名称的电子邮件到不同的收件人邮箱:
```python
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from email.mime.image import MIMEImage
import datetime
# 设置邮件服务器、用户名、密码等信息
smtp_server = 'smtp.example.com'
smtp_port = 587
smtp_user = 'your_username'
smtp_password = 'your_password'
# 定义收件人邮箱、邮件主题、邮件内容等信息
recipients = ['recipient1@example.com', 'recipient2@example.com']
mail_subject = 'Test Email'
mail_content = 'Hello, this is a test email sent by Python.'
# 创建邮件消息对象
msg = MIMEMultipart()
msg['Subject'] = mail_subject
msg['From'] = smtp_user
msg['To'] = ", ".join(recipients)
# 添加文本内容
text = MIMEText(mail_content)
msg.attach(text)
# 添加图片附件
with open('image.jpg', 'rb') as f:
img_data = f.read()
img = MIMEImage(img_data, name='image.jpg')
msg.attach(img)
# 发送邮件
try:
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls()
server.login(smtp_user, smtp_password)
for recipient in recipients:
msg['To'] = recipient
server.sendmail(smtp_user, recipient, msg.as_string())
print(f"Email sent to {recipient} at {datetime.datetime.now()}")
server.quit()
print("All emails sent successfully!")
except Exception as e:
print("Failed to send email:", e)
```
在上面的代码中,您需要将`smtp_server`、`smtp_port`、`smtp_user`和`smtp_password`替换为您自己的邮件服务器信息和邮箱登录信息。您还需要将`recipients`列表替换为您要发送电子邮件的收件人邮箱列表。如果您想发送不同的邮件内容,可以在代码中更改`mail_subject`和`mail_content`变量的值。如果您还想添加附件,则可以使用`MIMEImage`类添加图片附件,代码中的`image.jpg`是要添加的图片文件。
相关推荐
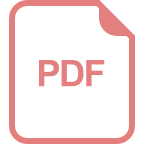
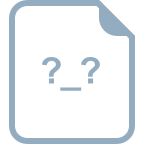
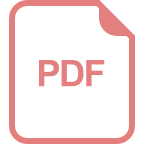
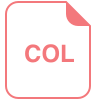













