能帮我 写一个C语言关于计算器的加减乘除和进制转换的代码吗
时间: 2023-08-28 18:32:20 浏览: 119
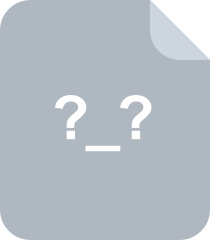
C语言编写的加减乘除运算
当然可以,以下是一个简单的C语言计算器和进制转换程序的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
int add(int a, int b) {
return a + b;
}
int subtract(int a, int b) {
return a - b;
}
int multiply(int a, int b) {
return a * b;
}
int divide(int a, int b) {
return a / b;
}
void convertToBinary(int n) {
if (n > 1) {
convertToBinary(n / 2);
}
printf("%d", n % 2);
}
void convertToOctal(int n) {
if (n > 7) {
convertToOctal(n / 8);
}
printf("%d", n % 8);
}
void convertToHexadecimal(int n) {
if (n > 15) {
convertToHexadecimal(n / 16);
}
int remainder = n % 16;
if (remainder < 10) {
printf("%d", remainder);
} else {
printf("%c", remainder - 10 + 'A');
}
}
int main() {
int num1, num2, choice;
char operation;
printf("Enter two numbers: ");
scanf("%d %d", &num1, &num2);
printf("Enter the operation (+, -, *, /): ");
scanf(" %c", &operation);
switch (operation) {
case '+':
printf("%d + %d = %d\n", num1, num2, add(num1, num2));
break;
case '-':
printf("%d - %d = %d\n", num1, num2, subtract(num1, num2));
break;
case '*':
printf("%d * %d = %d\n", num1, num2, multiply(num1, num2));
break;
case '/':
printf("%d / %d = %d\n", num1, num2, divide(num1, num2));
break;
default:
printf("Invalid operation!\n");
}
printf("Enter a number to convert to binary: ");
scanf("%d", &choice);
printf("%d in binary is: ", choice);
convertToBinary(choice);
printf("\n");
printf("Enter a number to convert to octal: ");
scanf("%d", &choice);
printf("%d in octal is: ", choice);
convertToOctal(choice);
printf("\n");
printf("Enter a number to convert to hexadecimal: ");
scanf("%d", &choice);
printf("%d in hexadecimal is: ", choice);
convertToHexadecimal(choice);
printf("\n");
return 0;
}
```
该程序使用了简单的函数来执行加法、减法、乘法、除法和进制转换,通过 switch 语句来决定执行哪种操作。在进制转换部分,程序使用递归函数来将一个整数转换为二进制、八进制或十六进制。
阅读全文
相关推荐


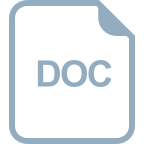




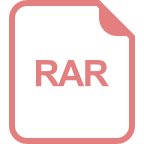
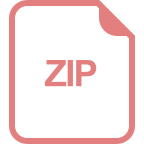
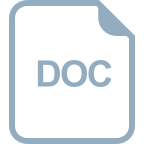




