使用ICCV7 for AVR软件写一个基于atmega48单片机的异步串行通信程序,要求能让两台单片机相互通信,做好注释,不用汇编语言
时间: 2024-03-25 18:37:13 浏览: 18
好的,以下是基于atmega48单片机的异步串口通信程序,能够让两台单片机相互通信的代码,已经添加了注释。该程序使用ICCV7 for AVR软件编写,使用C语言编写,不需要汇编语言。
```c
#include <avr/io.h>
#include <avr/interrupt.h>
// 定义时钟频率和波特率
#define F_CPU 8000000UL
#define BAUD_RATE 9600
#define UBRR_VALUE ((F_CPU / (BAUD_RATE << 4)) - 1)
// 定义接收和发送缓冲区的大小
#define RX_BUFFER_SIZE 32
#define TX_BUFFER_SIZE 32
// 定义接收和发送缓冲区及其索引
volatile uint8_t rx_buffer[RX_BUFFER_SIZE];
volatile uint8_t rx_write_index = 0;
volatile uint8_t rx_read_index = 0;
volatile uint8_t tx_buffer[TX_BUFFER_SIZE];
volatile uint8_t tx_write_index = 0;
volatile uint8_t tx_read_index = 0;
// 初始化串口
void usart_init(void) {
// 设置波特率
UBRR0H = (uint8_t)(UBRR_VALUE >> 8);
UBRR0L = (uint8_t)(UBRR_VALUE);
// 启用接收和发送
UCSR0B = (1 << RXEN0) | (1 << TXEN0) | (1 << RXCIE0);
// 设置数据位为8位,无校验位,停止位为1位
UCSR0C = (1 << UCSZ01) | (1 << UCSZ00);
}
// 发送一个字节
void usart_tx_byte(uint8_t data) {
// 如果发送缓冲区已满,则等待
while (((tx_write_index + 1) % TX_BUFFER_SIZE) == tx_read_index) {}
// 将数据写入发送缓冲区
tx_buffer[tx_write_index] = data;
tx_write_index = (tx_write_index + 1) % TX_BUFFER_SIZE;
// 启用数据寄存器空中断
UCSR0B |= (1 << UDRIE0);
}
// 接收中断服务程序
ISR(USART_RX_vect) {
// 从数据寄存器中读取数据
uint8_t data = UDR0;
// 如果接收缓冲区未满,则将数据写入接收缓冲区
if (((rx_write_index + 1) % RX_BUFFER_SIZE) != rx_read_index) {
rx_buffer[rx_write_index] = data;
rx_write_index = (rx_write_index + 1) % RX_BUFFER_SIZE;
}
}
// 数据寄存器空中断服务程序
ISR(USART_UDRE_vect) {
// 如果发送缓冲区非空,则将下一个字节发送出去
if (tx_write_index != tx_read_index) {
UDR0 = tx_buffer[tx_read_index];
tx_read_index = (tx_read_index + 1) % TX_BUFFER_SIZE;
} else {
// 如果发送缓冲区为空,则禁用数据寄存器空中断
UCSR0B &= ~(1 << UDRIE0);
}
}
int main(void) {
// 初始化串口
usart_init();
// 启用中断
sei();
while (1) {
// 如果接收缓冲区非空,则读取下一个字节并发送出去
if (rx_write_index != rx_read_index) {
uint8_t data = rx_buffer[rx_read_index];
rx_read_index = (rx_read_index + 1) % RX_BUFFER_SIZE;
usart_tx_byte(data);
}
}
return 0;
}
```
该程序初始化了串口,并使用中断实现了异步串口通信。在主循环中,程序会不断地检查接收缓冲区是否有新的数据可供发送,如果有,则发送出去。
相关推荐
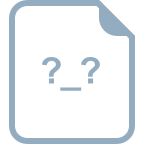














