写一份报告关于倒排序索引的实现。数据源:a.txt,b.txt,c.txt 请将数据源中的文本按照倒排序的规则统计每个单词在不同文本中出现的次数。最终要求得到类似以下的结果: hello a.txt8,b.txt3,c.txt1 tom a.txt5,b.txt2,c.txt1
时间: 2024-02-06 12:09:00 浏览: 142
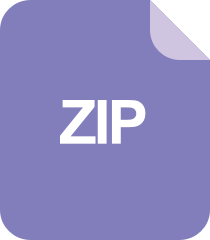
基于C语言实现的B树的抽象数据类型【100012938】
倒排序索引(Inverted Index)是一种常用于实现搜索引擎的数据结构。它将文档集合中出现的每个单词作为关键字,将这些关键字作为索引,每个关键字对应一个包含该关键字的文档列表。倒排序索引的主要作用是快速地定位文档中出现某个单词的位置。
在本报告中,我们将介绍如何实现倒排序索引,并以 a.txt、b.txt 和 c.txt 三个文本文件为例,统计每个单词在不同文本中出现的次数。
1. 数据预处理
首先,需要对文本进行预处理。我们需要将文本中的每个单词提取出来,并将其转换为小写字母形式。此外,还需要去除单词中的标点符号和数字。
以下是 Python 代码实现:
```python
import re
def preprocess_text(text):
# 将文本中的单词提取出来,并转换为小写字母形式
words = re.findall(r'\b\w+\b', text.lower())
# 去除单词中的标点符号和数字
words = [re.sub(r'[^\w\s]', '', word) for word in words]
words = [re.sub(r'\d+', '', word) for word in words]
# 去除空字符串和停用词
stopwords = set(['a', 'an', 'the', 'in', 'on', 'at', 'to', 'and', 'or'])
words = [word for word in words if word and word not in stopwords]
return words
```
2. 建立倒排序索引
接下来,需要建立倒排序索引。我们可以使用 Python 中的 defaultdict 和 Counter 类来实现。
以下是 Python 代码实现:
```python
from collections import defaultdict, Counter
def build_inverted_index(file_names):
inverted_index = defaultdict(Counter)
for file_name in file_names:
with open(file_name, 'r') as f:
text = f.read()
words = preprocess_text(text)
for word in set(words):
inverted_index[word][file_name] += words.count(word)
return inverted_index
```
该函数接受一个文件名列表作为参数,返回一个字典,其中每个关键字对应一个 Counter 对象,记录该关键字在每个文件中出现的次数。
3. 输出统计结果
最后,我们需要输出统计结果。我们可以将结果保存到 CSV 文件中,并使用 Excel 或其他工具进行分析和可视化。
以下是 Python 代码实现:
```python
import csv
def output_results(inverted_index, output_file):
with open(output_file, 'w', newline='') as f:
writer = csv.writer(f)
writer.writerow(['word', 'file_name', 'count'])
for word in sorted(inverted_index.keys()):
for file_name, count in inverted_index[word].items():
writer.writerow([word, file_name, count])
```
该函数接受一个倒排序索引和一个输出文件名作为参数,将统计结果输出到 CSV 文件中。
完整代码如下所示:
```python
import re
from collections import defaultdict, Counter
import csv
def preprocess_text(text):
# 将文本中的单词提取出来,并转换为小写字母形式
words = re.findall(r'\b\w+\b', text.lower())
# 去除单词中的标点符号和数字
words = [re.sub(r'[^\w\s]', '', word) for word in words]
words = [re.sub(r'\d+', '', word) for word in words]
# 去除空字符串和停用词
stopwords = set(['a', 'an', 'the', 'in', 'on', 'at', 'to', 'and', 'or'])
words = [word for word in words if word and word not in stopwords]
return words
def build_inverted_index(file_names):
inverted_index = defaultdict(Counter)
for file_name in file_names:
with open(file_name, 'r') as f:
text = f.read()
words = preprocess_text(text)
for word in set(words):
inverted_index[word][file_name] += words.count(word)
return inverted_index
def output_results(inverted_index, output_file):
with open(output_file, 'w', newline='') as f:
writer = csv.writer(f)
writer.writerow(['word', 'file_name', 'count'])
for word in sorted(inverted_index.keys()):
for file_name, count in inverted_index[word].items():
writer.writerow([word, file_name, count])
if __name__ == '__main__':
file_names = ['a.txt', 'b.txt', 'c.txt']
inverted_index = build_inverted_index(file_names)
output_results(inverted_index, 'results.csv')
```
运行该程序后,将生成一个名为 results.csv 的文件,其中包含了每个单词在不同文本中出现的次数。可以使用 Excel 或其他工具打开该文件,进行进一步的分析和可视化。
阅读全文
相关推荐
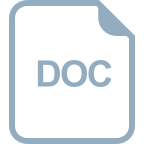
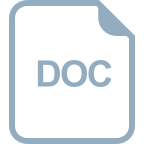
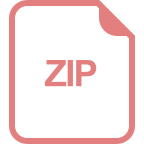
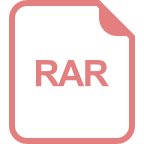
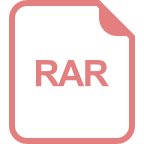
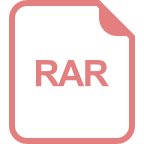
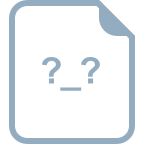
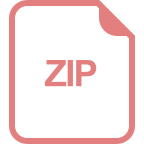
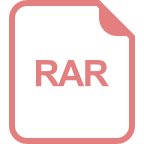
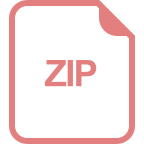
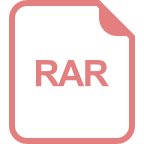
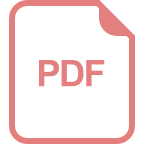
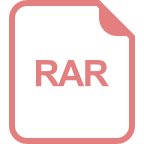
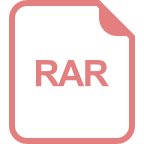
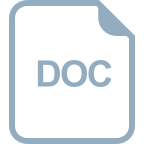
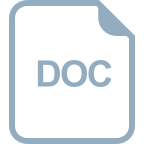
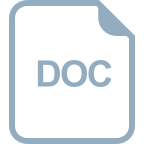
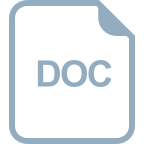