编写自定义函数total,统计一个字符串中字母、数字和其他字符的个数
时间: 2023-11-19 20:04:27 浏览: 50
可以使用Python内置的字符串方法isalpha、isdigit来判断字符的类型,进而统计个数。代码如下:
```python
def total(s):
alpha_count = digit_count = other_count = 0
for char in s:
if char.isalpha():
alpha_count += 1
elif char.isdigit():
digit_count += 1
else:
other_count += 1
return alpha_count, digit_count, other_count
```
使用示例:
```python
s = 'Hello, world! 123'
alpha_count, digit_count, other_count = total(s)
print(f"字母个数:{alpha_count}\n数字个数:{digit_count}\n其他字符个数:{other_count}")
```
输出结果:
```
字母个数:10
数字个数:3
其他字符个数:3
```
相关问题
编写自定义函数total,统计一个字符串中字母、数字和其他字符的个数。
可以使用Python的isalpha()、isdigit()函数来判断字符是字母还是数字,其他字符则为非字母或数字。
以下是实现函数的代码:
```python
def total(string):
alpha_count = 0 # 字母计数器
digit_count = 0 # 数字计数器
other_count = 0 # 其他字符计数器
for char in string:
if char.isalpha(): # 判断是否为字母
alpha_count += 1
elif char.isdigit(): # 判断是否为数字
digit_count += 1
else: # 非字母、数字即为其他字符
other_count += 1
return alpha_count, digit_count, other_count # 返回三种字符的个数元组
```
函数接受一个字符串作为参数,返回一个包含字母、数字和其他字符个数的元组。例如:
```python
string = 'Hello, world! 123'
print(total(string)) # 输出 (10, 3, 4)
```
其中,字符串中有10个字母、3个数字和4个其他字符。
c++编写自定义函数total,统计一个字符串中字母、数字和其他字符的个数。
可以使用C++的字符处理函数isalpha、isdigit等来判断字符是否为字母或数字,然后根据判断结果进行计数。以下是一个示例代码:
```c++
#include <iostream>
#include <string>
using namespace std;
void total(string str, int& alpha_count, int& digit_count, int& other_count) {
for (char c : str) {
if (isalpha(c)) {
alpha_count++;
} else if (isdigit(c)) {
digit_count++;
} else {
other_count++;
}
}
}
int main() {
string str = "Hello, World! 12345";
int alpha_count = 0;
int digit_count = 0;
int other_count = 0;
total(str, alpha_count, digit_count, other_count);
cout << "字母个数:" << alpha_count << endl;
cout << "数字个数:" << digit_count << endl;
cout << "其他字符个数:" << other_count << endl;
return 0;
}
```
输出结果为:
```
字母个数:10
数字个数:5
其他字符个数:4
```
阅读全文
相关推荐
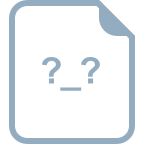









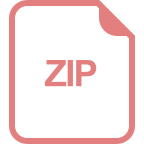