java 读取mysql数据库 并把数据封装成json文件
时间: 2024-02-12 11:05:25 浏览: 81
首先需要在Java中连接MySQL数据库,可以使用JDBC API实现。以下是一个连接MySQL数据库并查询数据的示例代码:
```
import java.sql.*;
public class MySQLDemo {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "root";
String password = "mypassword";
String query = "SELECT * FROM mytable";
try (Connection conn = DriverManager.getConnection(url, user, password);
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery(query)) {
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
// 将数据封装成JSON格式
String json = String.format("{\"id\": %d, \"name\": \"%s\", \"age\": %d}", id, name, age);
System.out.println(json);
}
} catch (SQLException e) {
System.out.println(e.getMessage());
}
}
}
```
上面的代码将查询结果封装成了JSON格式,可以根据需要将JSON数据写入文件中。以下是一个将JSON数据写入文件的示例代码:
```
import java.io.FileWriter;
import java.io.IOException;
public class JSONDemo {
public static void main(String[] args) {
String json = "{\"id\": 1, \"name\": \"John\", \"age\": 30}";
try (FileWriter writer = new FileWriter("output.json")) {
writer.write(json);
} catch (IOException e) {
System.out.println(e.getMessage());
}
}
}
```
将上面两段代码结合起来,就可以实现将MySQL数据库中的数据封装成JSON格式并写入文件的功能。
阅读全文
相关推荐
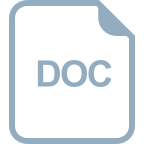
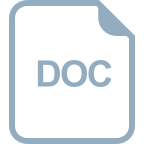
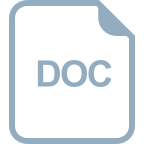
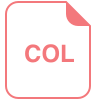
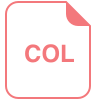
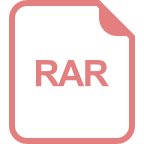
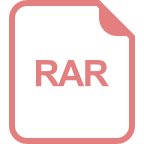
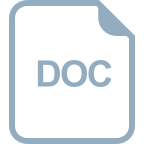
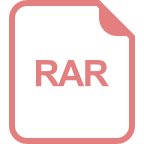
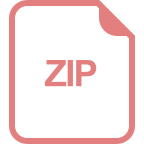
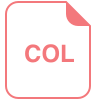
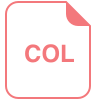
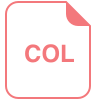
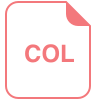



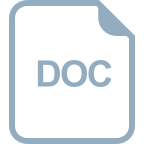