使用c++语言设计一个程序,用类 继承 派生
时间: 2024-02-24 22:00:15 浏览: 29
好的,我可以为您提供一个简单的示例程序。
我们可以创建一个基类Animal(动物),并从该类派生出两个子类Cat(猫)和Dog(狗)。每个类都有自己的属性和方法。
以下是示例代码:
```c++
#include <iostream>
using namespace std;
// Animal类
class Animal {
public:
string name;
int age;
void eat() {
cout << "Animal can eat." << endl;
}
void sleep() {
cout << "Animal can sleep." << endl;
}
};
// Cat类继承自Animal类
class Cat : public Animal {
public:
void meow() {
cout << "Cat can meow." << endl;
}
};
// Dog类继承自Animal类
class Dog : public Animal {
public:
void bark() {
cout << "Dog can bark." << endl;
}
};
int main() {
// 创建Cat对象
Cat cat;
cat.name = "Kitty";
cat.age = 2;
cat.eat();
cat.sleep();
cat.meow();
// 创建Dog对象
Dog dog;
dog.name = "Buddy";
dog.age = 3;
dog.eat();
dog.sleep();
dog.bark();
return 0;
}
```
在这个示例程序中,Animal是一个基类,它有两个属性(name和age)和两个方法(eat和sleep)。Cat和Dog都从Animal派生而来,并且都有自己的特定方法(meow和bark)。
在主函数中,我们创建了一个Cat对象和一个Dog对象,并访问它们的属性和方法。运行程序后,输出结果如下:
```
Animal can eat.
Animal can sleep.
Cat can meow.
Animal can eat.
Animal can sleep.
Dog can bark.
```
希望这个示例程序能够帮助您理解类的继承和派生。
相关推荐
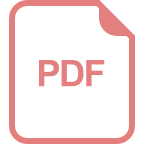














