使用 pyvista可视化带有RGB信息的ply文件的python代码
时间: 2024-06-10 08:04:55 浏览: 177
以下是使用 pyvista 库来可视化带有 RGB 信息的 PLY 文件的 Python 代码:
```
import pyvista as pv
import numpy as np
# Load PLY file with RGB scalar field
filename = 'your_filename.ply'
mesh = pv.read(filename)
# Extract RGB arrays
red_array = mesh.point_arrays['red']
green_array = mesh.point_arrays['green']
blue_array = mesh.point_arrays['blue']
rgb_array = np.column_stack((red_array, green_array, blue_array))
# Add RGB array as scalars
mesh['RGB'] = rgb_array
# Plot mesh with RGB information
p = pv.Plotter()
p.add_mesh(mesh, scalars='RGB')
p.show()
```
相关问题
可视化带有RGB信息的ply文件的python代码
以下是一个可视化带有RGB信息的PLY文件的简单Python代码示例:
```python
import open3d as o3d
# 加载带有RGB信息的PLY文件
mesh = o3d.io.read_triangle_mesh("example.ply")
pcd = mesh.vertices
pcd.colors = mesh.vertex_colors
# 可视化点云
o3d.visualization.draw_geometries([pcd])
```
这段代码使用Open3D库加载带有RGB信息的PLY文件,并将每个点的颜色设置为对应的RGB值。然后,它将点云可视化为一个窗口。注意,请替换文件名以匹配您自己的PLY文件。
彩色点云获取 python
在Python中,可以使用不同的库获取彩色点云数据。其中一种常用的库是Open3D。你可以使用Open3D库中的函数来读取彩色点云文件,并将其转换为点云数据结构。
首先,你需要安装Open3D库。可以使用pip命令进行安装,如下所示:
```
pip install open3d
```
接下来,你可以使用下面的代码来读取彩色点云文件:
```python
import open3d as o3d
# 读取彩色点云文件
pcd = o3d.io.read_point_cloud("path/to/your/file.ply")
# 查看点云信息
print(pcd)
```
在上面的代码中,你需要将"path/to/your/file.ply"替换为你的彩色点云文件的路径。
通过上述代码,你可以将彩色点云文件读取到一个点云数据结构中,并且可以查看点云的信息。你可以根据需要,对读取到的点云数据进行进一步的处理和操作,例如点云配准、点云分割、点云可视化等。在Open3D库中,还提供了许多函数和方法来进行这些操作。
请注意,上述代码只是一个简单的示例,实际应用中可能需要根据具体的彩色点云文件的格式和数据结构进行相应的调整。此外,在获取彩色点云之前,你可能需要先获取对应的深度图像和RGB图像数据,然后将它们组合成彩色点云。
引用:这里我们将配准好的点云文件直接拿过来用。这两个文件分别是bun001.ply文件和bun002.ply。文件下载地址为python点云拼接样例数据-深度学习文档类资源-CSDN下载。 1 python拼接程序 。
引用:python三维点云研究计划_Coding的叶子的博客-CSDN博客_3d点云 python将按照以下目录持续进行更新……点云格式介绍、点云可视化、点云投影、生成鸟瞰图、生成前视图、点云配准、点云分割、三维目标检测、点云重建、深度学习点云算法……https://blog.csdn.net/suiyingy/article/details/124017716点云配准(一)— ICP方法_Coding的叶子的博客-CSDN博客点云配准——ICP方法介绍https://blog.csdn.net/suiyingy/article/details/124336448更多三维、二维感知算法和金融量化分析算法请关注“乐乐感知学堂”微信公众号,并将持续进行更新。 。
引用:点云拼接主要是把不同的点云拼接到一起。通常,为了获得一个完整物体的三维点云,我们可能会在不同的视点进行数据采集,然后把采集的点云数据拼接到一起。 。 <span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [python点云拼接](https://blog.csdn.net/suiyingy/article/details/124343913)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
相关推荐
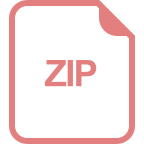
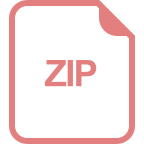
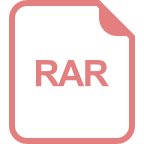
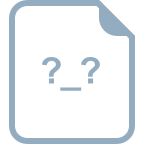
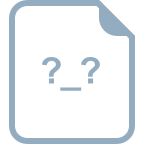
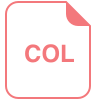



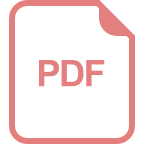
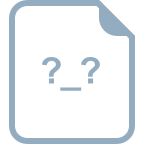