现TK界面中选择输入单张图片进行基于基于连通区域的字符分割方法分割单张图片多个数字以及图像预处理,处理过程显示并保存的界面设计,以完整的Python代码实现
时间: 2023-06-25 12:07:51 浏览: 34
以下是一个基于 TK 的界面设计,可以选择一张图片进行字符分割,同时进行图像预处理,并显示处理过程。最终结果可以保存。
```python
import tkinter as tk
from tkinter import filedialog
from PIL import Image, ImageTk
import cv2
import numpy as np
class Application(tk.Frame):
def __init__(self, master=None):
super().__init__(master)
self.master = master
self.pack()
self.create_widgets()
def create_widgets(self):
# 选择文件按钮
self.select_button = tk.Button(self, text="选择文件", command=self.select_image)
self.select_button.pack(side="top")
# 显示原图
self.original_label = tk.Label(self)
self.original_label.pack(side="left")
# 显示处理后的图像
self.processed_label = tk.Label(self)
self.processed_label.pack(side="right")
# 分割并保存图像按钮
self.split_button = tk.Button(self, text="分割并保存图像", command=self.split_and_save)
self.split_button.pack(side="bottom")
def select_image(self):
# 打开文件选择对话框
file_path = filedialog.askopenfilename(title="选择文件", filetypes=(("Image files", "*.jpg;*.jpeg;*.png"), ("All files", "*.*")))
if file_path:
# 将图像显示在界面上
image = Image.open(file_path)
self.original_image = image
self.processed_image = image.copy()
image = ImageTk.PhotoImage(image)
self.original_label.config(image=image)
self.original_label.image = image
def split_and_save(self):
if self.processed_image:
# 进行图像处理和字符分割
processed_image = np.array(self.processed_image)
gray_image = cv2.cvtColor(processed_image, cv2.COLOR_RGB2GRAY)
threshold_image = cv2.threshold(gray_image, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)[1]
kernel = np.ones((3,3), np.uint8)
dilated_image = cv2.dilate(threshold_image, kernel, iterations=1)
contours, hierarchy = cv2.findContours(dilated_image, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
bounding_boxes = [cv2.boundingRect(c) for c in contours]
bounding_boxes.sort(key=lambda x: x[0])
# 将分割后的字符保存为单独的图片
for i, box in enumerate(bounding_boxes):
x, y, w, h = box
digit_image = processed_image[y:y+h, x:x+w]
digit_image = Image.fromarray(digit_image)
digit_image.save(f"digit_{i}.png")
# 在界面上显示处理后的图像
processed_image = Image.fromarray(processed_image)
processed_image = ImageTk.PhotoImage(processed_image)
self.processed_label.config(image=processed_image)
self.processed_label.image = processed_image
def process_image(self):
# 进行图像预处理
if self.processed_image:
processed_image = np.array(self.processed_image)
gray_image = cv2.cvtColor(processed_image, cv2.COLOR_RGB2GRAY)
threshold_image = cv2.threshold(gray_image, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)[1]
kernel = np.ones((3,3), np.uint8)
processed_image = cv2.dilate(threshold_image, kernel, iterations=1)
self.processed_image = Image.fromarray(processed_image)
processed_image = ImageTk.PhotoImage(self.processed_image)
self.processed_label.config(image=processed_image)
self.processed_label.image = processed_image
root = tk.Tk()
app = Application(master=root)
app.mainloop()
```
在这个界面中,用户可以选择一张图片进行处理。点击“选择文件”按钮后,会弹出文件选择对话框。用户选择完毕后,原图会显示在界面左侧,并且会进行图像预处理,处理后的图像会显示在界面右侧。接着,用户可以点击“分割并保存图像”按钮,将处理后的图像进行字符分割,并保存为单独的图片。分割后的图像会显示在界面右侧,并可以保存至本地。
相关推荐
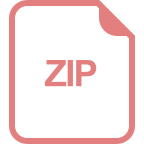
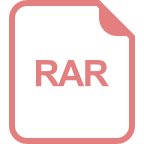






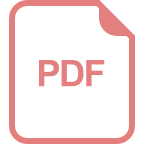
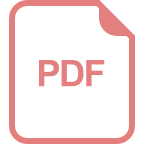
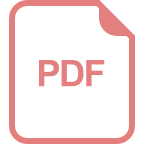
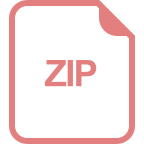
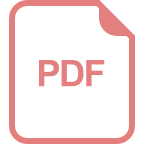
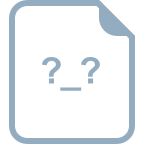
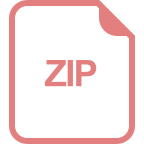
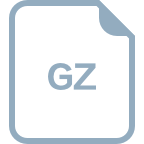
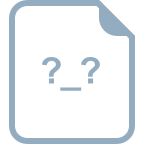