设计一个类模板,实现任意类型数组的排序(升序),至少包括整型、字符、字符串类型。
时间: 2024-03-13 13:43:59 浏览: 5
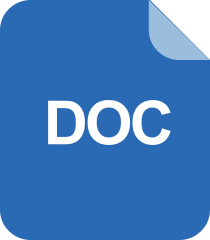
基于插入排序方法的类模板设计与实现.doc
好的,以下是一个示例类模板实现:
```cpp
#include <iostream>
#include <string>
template <typename T>
class SortArray {
public:
SortArray(T* arr, int size);
void sort();
void print();
private:
T* m_arr;
int m_size;
};
template <typename T>
SortArray<T>::SortArray(T* arr, int size) : m_arr(arr), m_size(size) {}
template <typename T>
void SortArray<T>::sort() {
for (int i = 0; i < m_size - 1; i++) {
for (int j = 0; j < m_size - i - 1; j++) {
if (m_arr[j] > m_arr[j + 1]) {
T temp = m_arr[j];
m_arr[j] = m_arr[j + 1];
m_arr[j + 1] = temp;
}
}
}
}
template <typename T>
void SortArray<T>::print() {
for (int i = 0; i < m_size; i++) {
std::cout << m_arr[i] << " ";
}
std::cout << std::endl;
}
int main() {
int intArr[] = {4, 2, 7, 1, 9};
SortArray<int> intSort(intArr, 5);
intSort.sort();
intSort.print();
char charArr[] = {'d', 'g', 'a', 'c', 'e'};
SortArray<char> charSort(charArr, 5);
charSort.sort();
charSort.print();
std::string strArr[] = {"apple", "banana", "orange", "pear", "kiwi"};
SortArray<std::string> strSort(strArr, 5);
strSort.sort();
strSort.print();
return 0;
}
```
这个类模板可以接受任何类型的数组,并实现升序排序。在 main 函数中,我们演示了如何使用该模板来排序整型、字符和字符串类型的数组。
阅读全文
相关推荐


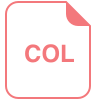
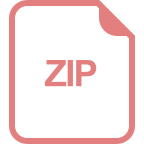
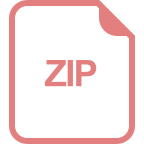
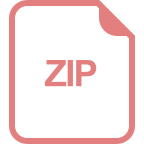
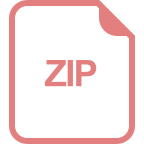
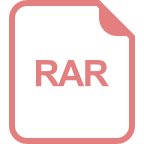
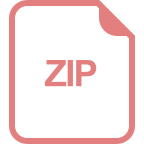
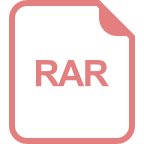