make a uart Verilog HDL program
时间: 2023-10-21 10:05:43 浏览: 141
As an AI language model, I cannot write Verilog HDL code, but I can provide a basic structure of a UART program in Verilog HDL.
In Verilog HDL, a UART module can be designed using the following basic structure:
1. Define the module and its input/output ports
```
module UART (
input clk,
input rst,
input txen,
input [7:0] txdata,
output reg tx,
output reg busy
);
```
2. Define the internal variables
```
reg [3:0] state;
reg [7:0] txbuf;
reg bitstart, bitstop;
reg [2:0] bitcnt;
```
3. Define the state machine for transmitting data
```
always @ (posedge clk, posedge rst) begin
if (rst) begin
state <= 0;
tx <= 1;
busy <= 0;
txbuf <= 0;
bitcnt <= 0;
bitstart <= 0;
bitstop <= 1;
end else begin
case (state)
0: begin // Wait for data to be ready
if (txen) begin
txbuf <= txdata;
state <= 1;
end
end
1: begin // Send start bit
tx <= 0;
bitstart <= 1;
bitcnt <= 0;
state <= 2;
end
2: begin // Send data bits
tx <= txbuf[bitcnt];
bitcnt <= bitcnt + 1;
if (bitcnt == 8) begin
state <= 3;
end
end
3: begin // Send stop bit
tx <= 1;
bitstop <= 0;
state <= 4;
end
4: begin // Wait for stop bit to finish
if (bitstop) begin
state <= 0;
busy <= 1;
end
end
default: begin
state <= 0;
tx <= 1;
busy <= 0;
txbuf <= 0;
bitcnt <= 0;
bitstart <= 0;
bitstop <= 1;
end
endcase
end
end
```
4. Define the initial values for the internal variables
```
initial begin
state <= 0;
tx <= 1;
busy <= 0;
txbuf <= 0;
bitcnt <= 0;
bitstart <= 0;
bitstop <= 1;
end
```
This is a basic structure for a UART program in Verilog HDL. The actual implementation may vary depending on the specific requirements and design constraints.
阅读全文
相关推荐









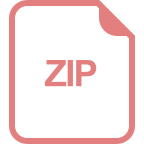




