(— 个月中的日期)编写一个程序,提示用户输人一个年份和一个月份名称的前三个字母(第一 个字母使用大写形式),显示该月中的天数。下面是一个运行示例java:
时间: 2023-06-08 15:08:16 浏览: 104
好的,下面是ChitGPT的回答:
您好,这是一个简单的Java程序。请问您需要的是Java代码还是Python代码呢?
如果是Java代码的话,可以用以下代码实现:
import java.util.Scanner;
public class MonthDays {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("请输入一个年份:");
int year = input.nextInt();
System.out.print("请输入一个月份的前三个字母(第一个字母使用大写形式): ");
String month = input.next();
// 创建一个记录每月天数的数组,其中二月默认为28天
int[] daysInMonths = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
// 判断闰年并修改二月天数为29天
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
daysInMonths[1] = 29;
}
// 根据输入的月份名称输出该月中的天数
switch (month) {
case "Jan":
System.out.println("31天");
break;
case "Feb":
System.out.println(daysInMonths[1] + "天");
break;
case "Mar":
System.out.println("31天");
break;
case "Apr":
System.out.println("30天");
break;
case "May":
System.out.println("31天");
break;
case "Jun":
System.out.println("30天");
break;
case "Jul":
System.out.println("31天");
break;
case "Aug":
System.out.println("31天");
break;
case "Sep":
System.out.println("30天");
break;
case "Oct":
System.out.println("31天");
break;
case "Nov":
System.out.println("30天");
break;
case "Dec":
System.out.println("31天");
break;
default:
System.out.println("输入的月份有误!");
}
}
}
如果是Python代码的话,可以用以下代码实现:
# -*- coding: utf-8 -*-
def leap_year(year):
return (year % 4 == 0 and year % 100 != 0) or year % 400 == 0
def month_days(year, month_name):
days_in_months = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
# 判断闰年并修改二月天数为29天
if leap_year(year):
days_in_months[1] = 29
# 根据输入的月份名称输出该月中的天数
if month_name == 'Jan':
return '31天'
elif month_name == 'Feb':
return str(days_in_months[1]) + '天'
elif month_name == 'Mar':
return '31天'
elif month_name == 'Apr':
return '30天'
elif month_name == 'May':
return '31天'
elif month_name == 'Jun':
return '30天'
elif month_name == 'Jul':
return '31天'
elif month_name == 'Aug':
return '31天'
elif month_name == 'Sep':
return '30天'
elif month_name == 'Oct':
return '31天'
elif month_name == 'Nov':
return '30天'
elif month_name == 'Dec':
return '31天'
else:
return '输入的月份有误!'
# 主程序
def main():
year = int(input('请输入一个年份:'))
month_name = input('请输入一个月份的前三个字母(第一个字母使用大写形式):')
result = month_days(year, month_name)
print(result)
if __name__ == '__main__':
main()
相关推荐
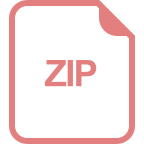
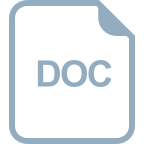
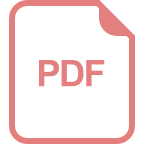











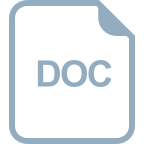
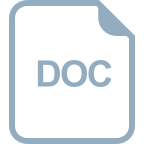
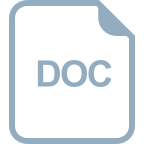
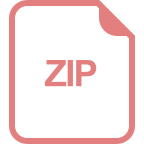