那么用opencv该怎么识别一个字幕是固定字幕还是普通字幕还是滚动字幕呢?请用C++写一个判别这三种字幕的代码
时间: 2024-05-10 21:14:54 浏览: 231
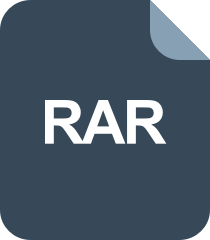
视频字幕解析程序,C++

要判别一个字幕是固定字幕还是普通字幕还是滚动字幕,可以考虑使用 OpenCV 中的文本检测和识别功能。下面是一个简单的示例代码,其中包含了如何使用 OpenCV 检测文本区域、如何获取文本区域的坐标、如何识别文本内容以及如何根据文本区域的位置和大小来判断字幕的类型。这里只是一个简单的示例,实际应用中需要根据具体的场景和需求进行调整和优化。
```c
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 加载图像
Mat image = imread("subtitles.png");
// 将图像转换为灰度图像
Mat gray;
cvtColor(image, gray, COLOR_BGR2GRAY);
// 对图像进行二值化处理
Mat binary;
threshold(gray, binary, 0, 255, THRESH_OTSU);
// 进行文本检测
Ptr<text::OCRBeamSearchDecoder> ocr = text::OCRHolisticWordRecognizer::create();
vector<Rect> boxes;
vector<string> words;
vector<float> confidences;
ocr->run(image, binary, boxes, words, confidences, OCR_LEVEL_WORD);
// 遍历文本区域,根据位置和大小判断字幕类型
for (int i = 0; i < boxes.size(); i++)
{
Rect box = boxes[i];
string word = words[i];
// 计算文本区域的宽度和高度
int width = box.width;
int height = box.height;
// 根据宽度和高度判断字幕类型
if (width > image.cols * 0.8 && height > image.rows * 0.8)
{
cout << "固定字幕:" << word << endl;
}
else if (width > image.cols * 0.8)
{
cout << "普通字幕:" << word << endl;
}
else
{
cout << "滚动字幕:" << word << endl;
}
}
return 0;
}
```
需要注意的是,这里使用了 OCRBeamSearchDecoder 和 OCRHolisticWordRecognizer 进行文本检测和识别,需要安装 OpenCV 的文本识别模块(text)才能使用。具体安装方法可以参考 OpenCV 的官方文档。
阅读全文
相关推荐
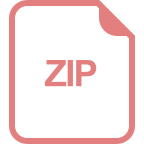
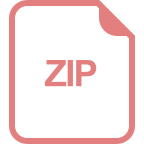
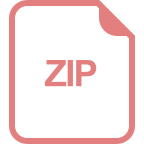
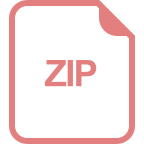
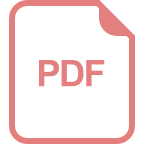
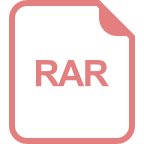
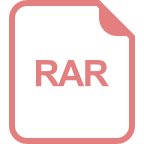
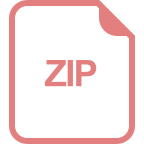
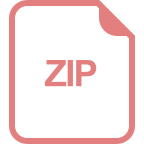
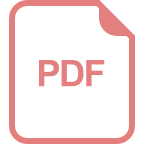
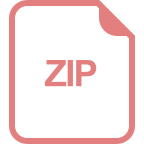
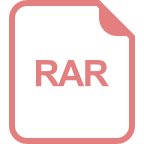