Java语言写一个读取本地excal表中的数据并写入数据库的方法
时间: 2024-05-14 07:14:09 浏览: 81
以下是一个简单的Java程序,用于读取本地Excel表中的数据并将其写入数据库。这个程序使用Apache POI库来读取Excel表中的数据,并使用JDBC连接数据库并将数据写入其中。
请注意,此代码仅供参考,需要根据实际情况进行修改和调整。
```java
import java.io.File;
import java.io.FileInputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.util.Iterator;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelToDatabase {
public static void main(String[] args) {
String jdbcUrl = "jdbc:mysql://localhost:3306/mydatabase";
String username = "myusername";
String password = "mypassword";
String excelFilePath = "C:/data/myexcel.xlsx";
try (Connection connection = DriverManager.getConnection(jdbcUrl, username, password)) {
FileInputStream inputStream = new FileInputStream(new File(excelFilePath));
XSSFWorkbook workbook = new XSSFWorkbook(inputStream);
Sheet sheet = workbook.getSheetAt(0);
Iterator<Row> iterator = sheet.iterator();
String sql = "INSERT INTO mytable (col1, col2, col3) VALUES (?, ?, ?)";
PreparedStatement statement = connection.prepareStatement(sql);
int count = 0;
while (iterator.hasNext()) {
Row nextRow = iterator.next();
Iterator<Cell> cellIterator = nextRow.cellIterator();
while (cellIterator.hasNext()) {
Cell nextCell = cellIterator.next();
int columnIndex = nextCell.getColumnIndex();
switch (columnIndex) {
case 0:
statement.setString(1, nextCell.getStringCellValue());
break;
case 1:
statement.setString(2, nextCell.getStringCellValue());
break;
case 2:
statement.setDouble(3, nextCell.getNumericCellValue());
break;
}
}
statement.addBatch();
if (count % 20 == 0) {
statement.executeBatch();
}
}
workbook.close();
statement.executeBatch();
System.out.println("Data has been inserted into database successfully.");
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在这个程序中,我们首先声明了要使用的JDBC连接和Excel文件的路径。然后我们使用Apache POI库中的XSSFWorkbook类来读取Excel文件。我们遍历工作表中的每一行并使用PreparedStatement对象将数据插入到数据库中。我们使用addBatch()方法来批量插入数据,这样可以提高性能。最后,我们关闭工作簿并在控制台上打印成功消息。
请注意,此代码仅供参考,需要根据实际情况进行修改和调整。特别是在处理不同类型的单元格数据时,需要根据实际情况进行修改。
阅读全文
相关推荐



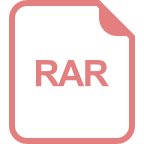




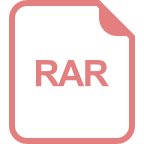


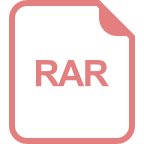






