vue在向后端发送请求时 如何在cookie上再添加一个参数
时间: 2024-04-15 18:26:13 浏览: 15
在 Vue 中发送请求时,可以通过在请求头中添加自定义的参数来实现在 cookie 上再添加一个参数。你可以使用 Axios 库作为示例来说明:
```javascript
axios.get('https://api.example.com/data', {
headers: {
Cookie: 'your_cookie_value',
'X-Custom-Param': 'your_custom_param_value'
}
})
.then(response => {
// 处理响应
})
.catch(error => {
// 处理错误
});
```
在上面的例子中,使用了 Axios 库发送 GET 请求,并在请求头中通过 `headers` 选项设置了 Cookie 和自定义的参数 `X-Custom-Param`。你可以根据实际情况设置正确的参数名和值。
如果你需要在多个请求中都添加这个自定义参数,可以考虑封装一个请求拦截器,在拦截器中统一处理这个参数,避免在每个请求中重复设置。
请注意,确保自定义参数的命名不会与其他已有的请求头字段冲突。此外,还要遵守后端接口的要求,确保后端能够正确解析和处理这个自定义参数。
这只是其中一种实现方式,具体实现方法可能因项目配置和需求而有所不同。
相关问题
java vue怎么在后端配置跨域
在后端配置跨域,可以通过 Spring Boot 和 Vue.js 的组合进行实现。
下面是一个简单的 Spring Boot 配置跨域的示例:
```java
@Configuration
public class CorsConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("*")
.allowedMethods("*")
.allowedHeaders("*")
.allowCredentials(true)
.maxAge(3600);
}
}
```
上述代码中,`@Configuration` 注解表示这是一个配置类,`CorsConfig` 类实现了 `WebMvcConfigurer` 接口。在 `addCorsMappings` 方法中,通过调用 `CorsRegistry` 对象的方法来配置跨域。
具体来说,`.addMapping("/**")` 表示配置所有的请求都支持跨域访问,`.allowedOrigins("*")` 表示所有的来源都可以访问,`.allowedMethods("*")` 表示所有的方法都可以访问,`.allowedHeaders("*")` 表示所有的头信息都可以访问,`.allowCredentials(true)` 表示允许发送 Cookie,`.maxAge(3600)` 表示缓存时间为 3600 秒。
在 Vue.js 中,可以通过在 `vue.config.js` 文件中配置代理来实现跨域。
以下是一个示例代码:
```javascript
module.exports = {
devServer: {
proxy: {
'/api': {
target: 'http://localhost:8080',
changeOrigin: true,
pathRewrite: {
'^/api': ''
}
}
}
}
}
```
以上代码中,`'/api'` 表示需要代理的路径,`target: 'http://localhost:8080'` 表示代理到的地址,`changeOrigin: true` 表示开启跨域,`pathRewrite` 表示重写路径。在 Vue.js 中发送请求时,只需要将请求路径的前缀改为 `/api` 即可,例如 `/api/user`。这样就可以实现跨域访问了。
vue请求头携带的cookie换了一个环境就没有了
你好!对于 Vue 请求携带的 cookie,换了一个环境后没有了的问题,可能是由于跨域请求导致的。浏览器默认情况下,跨域请求是不携带 cookie 的,为了解决这个问题,你可以尝试以下方法:
1. 后端设置允许跨域请求携带 cookie。在后端接口的响应头中添加以下字段:
```
Access-Control-Allow-Origin: <your_domain>
Access-Control-Allow-Credentials: true
```
`<your_domain>` 是你的前端域名或 IP 地址。这样设置后,浏览器会允许跨域请求携带 cookie。
2. 前端请求中设置 `withCredentials` 为 `true`。在 Vue 的请求中添加以下配置:
```javascript
axios.defaults.withCredentials = true;
```
或者在每个请求中单独设置:
```javascript
axios.get(url, { withCredentials: true });
```
这样设置后,浏览器会在跨域请求中携带 cookie。
请注意,确保后端接口正确设置跨域响应头,并且前端请求中设置了 `withCredentials` 字段。同时,还要注意前端和后端的域名和端口是否匹配,否则仍然可能出现跨域问题。
希望以上方法能帮到你!如果还有其他问题,请随时提问。
相关推荐
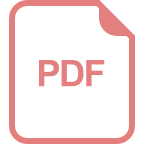
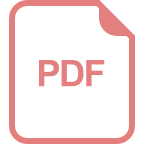
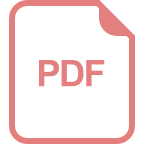












