怎样将C++string中的一段字符用另外的字符替换
时间: 2023-02-16 08:03:39 浏览: 59
在C语言中,可以使用字符数组和循环语句来实现字符串中一段字符的替换。
举个例子,假设我们要将字符串 "Hello World" 中所有的字符 "o" 替换成字符 "x" ,可以使用如下代码:
```
#include <stdio.h>
int main()
{
char str[] = "Hello World";
int i;
for (i = 0; str[i] != '\0'; i++)
{
if (str[i] == 'o')
{
str[i] = 'x';
}
}
printf("%s\n", str);
return 0;
}
```
输出结果:
```
Hellx Wxrld
```
代码中我们使用了一个循环语句来遍历字符串中的每一个字符,并在当前字符为 "o" 时将其替换为 "x"。
相关问题
c++ 如何替换string 字符串中的某段内容
可以使用`std::string`类的`replace()`函数来替换字符串中的某段内容。`replace()`函数的参数包括要替换的起始位置、要替换的字符数量和替换后的字符串。以下是一个示例:
```c++
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
std::string newStr = "everyone";
// 从索引6处开始,替换5个字符为"everyone"
str.replace(6, 5, newStr);
std::cout << str << std::endl; // 输出:Hello, everyone!
return 0;
}
```
在上面的示例中,`replace()`函数将字符串中从索引6处开始的5个字符替换为"everyone"。
用c++写一段替换字符的代码
### 回答1:
示例:#include <stdio.h>
int main()
{
char str[100];
char c1, c2;
printf("请输入字符串:");
gets(str);
printf("请输入要替换的字符:");
scanf("%c", &c1);
getchar();
printf("请输入替换后的字符:");
scanf("%c", &c2);
for(int i = 0; i < 100; i++)
{
if(str[i] == c1)
str[i] = c2;
}
printf("替换后的字符串:%s\n", str);
return 0;
}
### 回答2:
下面是一段用C语言编写的替换字符的代码:
```c
#include <stdio.h>
#include <string.h>
void replaceChar(char *str, char oldChar, char newChar) {
int length = strlen(str);
for (int i = 0; i < length; i++) {
if (str[i] == oldChar) {
str[i] = newChar;
}
}
}
int main() {
char str[100];
char oldChar, newChar;
printf("请输入一个字符串:");
fgets(str, sizeof(str), stdin);
printf("请输入要替换的字符:");
scanf("%c", &oldChar);
getchar(); // 需要读取多余的回车符
printf("请输入替换后的字符:");
scanf("%c", &newChar);
replaceChar(str, oldChar, newChar);
printf("替换后的字符串为:%s", str);
return 0;
}
```
这段代码定义了一个`replaceChar`函数,接受三个参数:字符串指针、要替换的字符和替换后的字符。函数通过遍历字符串的每个字符,如果遇到要替换的字符,则将其替换为新的字符。
在`main`函数中,首先接受用户输入的字符串,然后分别接受用户输入的要替换的字符和替换后的字符。接着调用`replaceChar`函数进行替换操作,并输出替换后的字符串。
### 回答3:
下面是一个用C语言编写的替换字符的代码示例:
```c
#include <stdio.h>
void replaceChar(char* str, char find, char replace) {
char* p = str;
while (*p != '\0') {
if (*p == find) {
*p = replace;
}
p++;
}
}
int main() {
char str[100];
char find, replace;
printf("请输入字符串: ");
fgets(str, sizeof(str), stdin);
printf("请输入要替换的字符: ");
scanf("%c", &find);
getchar(); // 清除输入缓冲区的换行符
printf("请输入替换后的字符: ");
scanf("%c", &replace);
replaceChar(str, find, replace);
printf("替换后的字符串为:%s", str);
return 0;
}
```
这段代码中定义了一个名为`replaceChar`的函数,用于将字符串中的某个字符替换为另一个字符。在`main`函数中,我们首先输入待替换的字符串,然后输入要替换的字符和替换后的字符。最后调用`replaceChar`函数进行字符的替换,并输出替换后的字符串。
注意,此代码假设字符串长度不超过100个字符。如果字符串长度超过100个字符,需要相应地增加数组`str`的大小。
相关推荐
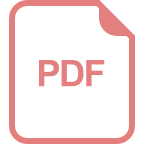
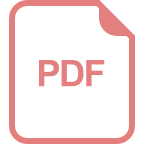
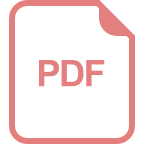












