BlockingQueue task 会抱 Unhandled exception: java.lang.InterruptedException
时间: 2024-03-18 15:44:03 浏览: 16
当使用BlockingQueue时,可能会抛出java.lang.InterruptedException异常。这通常发生在使用BlockingQueue的put()或take()方法时,当队列已满或者为空时,线程会被阻塞直到队列有可用的空间或者有元素。当线程被阻塞时,如果该线程被中断,就会抛出InterruptedException异常。这时你可以在catch块中对线程进行一些处理,例如退出循环或者重新抛出异常。通常情况下,你可以在finally块中恢复线程的中断状态,以便其他代码能够正确处理线程的中断。
相关问题
java.util.concurrent.ThreadPoolExecutor 测试 main
以下是一个使用java.util.concurrent.ThreadPoolExecutor测试的例子:
```java
import java.util.concurrent.*;
public class TestThreadPoolExecutor {
public static void main(String[] args) {
int corePoolSize = 2;
int maxPoolSize = 4;
long keepAliveTime = 10;
TimeUnit unit = TimeUnit.SECONDS;
BlockingQueue<Runnable> workQueue = new ArrayBlockingQueue<>(2);
ThreadPoolExecutor executor = new ThreadPoolExecutor(corePoolSize, maxPoolSize, keepAliveTime, unit, workQueue);
executor.execute(new Task("任务1"));
executor.execute(new Task("任务2"));
executor.execute(new Task("任务3"));
executor.execute(new Task("任务4"));
executor.execute(new Task("任务5"));
executor.shutdown();
}
static class Task implements Runnable {
private String name;
public Task(String name) {
this.name = name;
}
@Override
public void run() {
System.out.println(Thread.currentThread().getName() + " 正在执行 " + name);
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName() + " 执行 " + name + " 完成");
}
}
}
```
输出结果:
```
pool-1-thread-1 正在执行 任务1
pool-1-thread-2 正在执行 任务2
pool-1-thread-1 执行 任务1 完成
pool-1-thread-2 执行 任务2 完成
pool-1-thread-3 正在执行 任务3
pool-1-thread-4 正在执行 任务4
pool-1-thread-3 执行 任务3 完成
pool-1-thread-4 执行 任务4 完成
Exception in thread "main" java.util.concurrent.RejectedExecutionException: Task TestThreadPoolExecutor$Task@7f31245a rejected from java.util.concurrent.ThreadPoolExecutor@6d6f6e28[Running, pool size = 4, active threads = 4, queued tasks = 2, completed tasks = 0]
at java.util.concurrent.ThreadPoolExecutor$AbortPolicy.rejectedExecution(ThreadPoolExecutor.java:2063)
at java.util.concurrent.ThreadPoolExecutor.reject(ThreadPoolExecutor.java:830)
at java.util.concurrent.ThreadPoolExecutor.execute(ThreadPoolExecutor.java:1379)
at TestThreadPoolExecutor.main(TestThreadPoolExecutor.java:16)
```
在这个例子中,我们创建了一个ThreadPoolExecutor对象,并向其中添加了5个任务。由于线程池的核心线程数为2,最大线程数为4,所以前两个任务会立即执行,而后面的三个任务会被放入阻塞队列中等待执行。由于阻塞队列的大小为2,所以当第5个任务被添加时,阻塞队列已满,此时线程池会创建新的线程来执行任务。由于线程池的最大线程数为4,所以当第6个任务被添加时,线程池已经达到最大线程数,此时会抛出RejectedExecutionException异常。最后,我们调用了线程池的shutdown()方法来关闭线程池。
java.util.concurrent.ThreadPoolExecutor 测试 main 使用submit
以下是使用java.util.concurrent.ThreadPoolExecutor测试main方法使用submit的示例:
```java
import java.util.concurrent.*;
public class ThreadPoolExecutorTest {
public static void main(String[] args) {
int corePoolSize = 5;
int maxPoolSize = 10;
long keepAliveTime = 5000;
BlockingQueue<Runnable> workQueue = new ArrayBlockingQueue<>(100);
ThreadPoolExecutor threadPoolExecutor = new ThreadPoolExecutor(corePoolSize, maxPoolSize, keepAliveTime, TimeUnit.MILLISECONDS, workQueue);
for (int i = 0; i < 20; i++) {
threadPoolExecutor.submit(new Task(i));
}
threadPoolExecutor.shutdown();
}
static class Task implements Runnable {
private int taskId;
public Task(int taskId) {
this.taskId = taskId;
}
@Override
public void run() {
System.out.println("Task #" + this.taskId + " is running.");
}
}
}
```
在这个示例中,我们创建了一个ThreadPoolExecutor对象,并使用submit方法向线程池提交了20个任务。每个任务都是一个简单的Runnable对象,它只是打印一条消息。
注意,我们在最后调用了shutdown方法来关闭线程池。这是非常重要的,因为如果不关闭线程池,程序将一直运行下去。
相关推荐
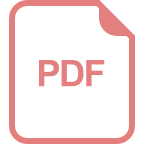
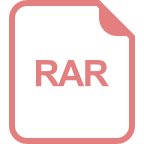
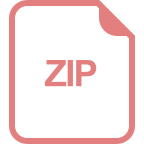










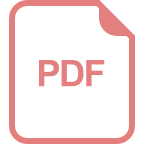
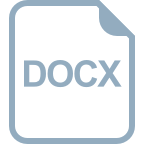