java如果使用Apache POI把word转pdf,提供代码示例
时间: 2023-08-31 08:28:25 浏览: 87
### 回答1:
在使用 Apache POI 转换 Word 文档为 PDF 文件之前,您需要在系统中安装 Apache POI 库并将其导入到您的项目中。
示例代码如下:
```
import java.io.FileInputStream;
import java.io.FileOutputStream;
import org.apache.poi.xwpf.converter.pdf.PdfConverter;
import org.apache.poi.xwpf.converter.pdf.PdfOptions;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
public class WordToPDFConverter {
public static void main(String[] args) throws Exception {
// 读取 Word 文档
FileInputStream inputStream = new FileInputStream("input.docx");
XWPFDocument document = new XWPFDocument(inputStream);
// 准备转换为 PDF
FileOutputStream out = new FileOutputStream("output.pdf");
PdfOptions options = PdfOptions.create();
// 转换为 PDF
PdfConverter.getInstance().convert(document, out, options);
// 关闭输入输出流
out.close();
inputStream.close();
}
}
```
在这段代码中,我们首先读取了名为 "input.docx" 的 Word 文档,然后使用 PdfConverter 将其转换为 PDF 格式并写入名为 "output.pdf" 的文件中。
### 回答2:
使用Apache POI库将Word文档转换为PDF文件,以下是示例代码:
```java
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFRun;
import org.apache.poi.xwpf.usermodel.XWPFTable;
import org.apache.poi.xwpf.usermodel.XWPFTableCell;
import org.apache.poi.xwpf.usermodel.XWPFTableRow;
import org.apache.poi.xwpf.usermodel.XWPFPicture;
import org.apache.poi.util.IOUtils;
import org.apache.poi.wp.usermodel.HeaderFooterType;
import org.apache.poi.xwpf.converter.pdf.PdfConverter;
import org.apache.poi.xwpf.converter.pdf.PdfOptions;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.OutputStream;
public class WordToPdfConverter {
public static void main(String[] args) {
try {
String wordFilePath = "path/to/word.docx";
String pdfFilePath = "path/to/output.pdf";
FileInputStream fileInputStream = new FileInputStream(new File(wordFilePath));
XWPFDocument document = new XWPFDocument(fileInputStream);
// 设置PDF选项
PdfOptions options = PdfOptions.create();
// 创建输出流
OutputStream out = new FileOutputStream(new File(pdfFilePath));
// 将Word文档转换为PDF
PdfConverter.getInstance().convert(document, out, options);
// 关闭流
out.close();
document.close();
System.out.println("Word转PDF文件成功!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
以上代码使用了Apache POI的`XWPFDocument`类来加载Word文档,创建了一个`PdfOptions`对象来设置PDF选项。然后使用`PdfConverter`类的`convert`方法将Word文档转换为PDF文件,并保存到指定位置的文件。最后关闭相关的流和文档对象。
### 回答3:
使用Apache POI将Word转换为PDF需要借助iText库。下面是一个简单的示例代码:
```
import org.apache.poi.xwpf.converter.pdf.PdfConverter;
import org.apache.poi.xwpf.converter.pdf.PdfOptions;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import java.io.*;
public class WordToPdfConverter {
public static void main(String[] args) {
String inputFilePath = "input.docx";
String outputFilePath = "output.pdf";
FileInputStream inputFile = null;
FileOutputStream outputFile = null;
try {
inputFile = new FileInputStream(inputFilePath);
outputFile = new FileOutputStream(outputFilePath);
XWPFDocument document = new XWPFDocument(inputFile);
PdfOptions options = PdfOptions.create();
PdfConverter.getInstance().convert(document, outputFile, options);
System.out.println("Word转PDF成功!");
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (inputFile != null) {
inputFile.close();
}
if (outputFile != null) {
outputFile.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
在上述示例中,我们首先指定了输入文件路径(input.docx)和输出文件路径(output.pdf)。然后,我们使用FileInputStream从输入文件中读取Word文档,并使用FileOutputStream将转换后的PDF写入输出文件。
接下来,我们创建XWPFDocument对象,该对象代表了Word文档。然后,我们通过PdfConverter.getInstance().convert将Word文档转换为PDF,并使用PdfOptions.create创建转换选项。
最后,我们在控制台打印出转换成功的消息,并关闭输入和输出流。
需要注意的是,使用Apache POI将Word转换为PDF需要添加相关依赖包。可以在项目的构建工具(如Maven或Gradle)的配置文件中添加如下依赖:
```
<!-- Apache POI -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<!-- Apache POI - OOXML -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
<!-- iText -->
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.5.13.2</version>
</dependency>
```
以上是一个基本的示例代码,可以根据具体需求进行进一步的修改和扩展。
相关推荐
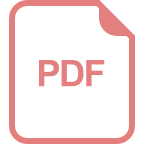
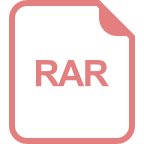













