threading库线程守护
时间: 2023-07-07 19:20:38 浏览: 92
好的,您的问题是关于Python的threading库中线程守护的问题。在Python中,每个线程都有一个守护标志(daemon flag),默认情况下这个标志是False,也就是说当主线程退出时,所有子线程仍然会继续执行直到它们完成或者被强制停止。如果将一个线程的守护标志设置为True,那么当主线程退出时,这个线程会立即停止执行,无论它是否完成。这个特性非常适合在程序结束时自动清理资源或者进行一些清理工作。在Python中,可以通过Thread类的daemon属性来设置一个线程的守护标志,例如:
```python
import threading
def worker():
while True:
print("Worker is running...")
t = threading.Thread(target=worker)
t.daemon = True
t.start()
```
在这个例子中,我们创建了一个名为worker的线程,并将它的守护标志设置为True。当主线程退出时,worker线程会立即停止运行。
相关问题
python中threading关闭线程
### 回答1:
在Python中,可以使用threading模块来创建和管理线程。要关闭一个线程,可以使用Thread对象的方法之一——setDaemon()。将线程的daemon标志设置为True,可以将线程设置为守护线程,这意味着当主线程退出时,它将自动关闭。例如:
```
import threading
import time
def worker():
print("Starting worker thread")
time.sleep(5)
print("Exiting worker thread")
t = threading.Thread(target=worker)
t.setDaemon(True) # 将线程设置为守护线程
t.start()
print("Main thread exiting")
```
在上面的示例中,worker线程被设置为守护线程,因此当主线程退出时,它将自动关闭。如果不将线程设置为守护线程,则必须手动关闭线程,例如:
```
import threading
import time
def worker():
print("Starting worker thread")
time.sleep(5)
print("Exiting worker thread")
t = threading.Thread(target=worker)
t.start()
time.sleep(2) # 等待2秒钟
t._stop() # 关闭线程
print("Main thread exiting")
```
在上面的示例中,worker线程将在5秒钟后退出。但是,我们在等待2秒钟后手动关闭了线程。请注意,这种方法不是很安全,因为它可能会导致线程在执行某些操作时被强制终止。因此,最好使用setDaemon()方法来关闭线程。
### 回答2:
在Python中关闭线程的方式主要有两种:通过设置标志位或使用线程对象的方法。
第一种方式是通过设置标志位来控制线程结束。在需要结束线程时,可以通过设置一个变量或标志位来通知线程退出循环,达到结束线程的目的。例如:
```python
import threading
import time
class MyThread(threading.Thread):
def __init__(self):
threading.Thread.__init__(self)
self.stop_event = threading.Event()
def run(self):
while not self.stop_event.is_set():
print("Thread is running...")
time.sleep(1)
def stop(self):
self.stop_event.set()
t = MyThread()
t.start()
time.sleep(5)
t.stop()
```
在上面的代码中,我们通过一个名为 stop_event 的 threading.Event() 对象来控制线程是否退出循环。在 run() 方法中,我们使用了 is_set() 方法检查标志位是否被设置为 True,如果是,则退出循环并结束线程。
第二种方式是使用线程对象的方法来结束线程。Python中的线程对象提供了一个 stop() 方法用于结束线程。但是,由于这个方法在Python2中被废弃,在Python3中也已经不存在了。因此,我们需要自己实现一个 stop() 方法来结束线程。一个简单的例子如下:
```python
import threading
import time
class MyThread(threading.Thread):
def __init__(self):
threading.Thread.__init__(self)
self.running = True
def run(self):
while self.running:
print("Thread is running...")
time.sleep(1)
def stop(self):
self.running = False
t = MyThread()
t.start()
time.sleep(5)
t.stop()
```
在上面的代码中,我们通过设置一个 boolean 类型的变量 running 来控制线程是否结束。在 stop() 方法中,我们将 running 设置为 False,从而结束线程的运行。需要注意的是,不推荐使用这种方法来结束线程,因为它可能会导致线程在执行关键代码时被意外打断,从而导致程序出错或崩溃。
### 回答3:
Python中的线程(Thread)是一种轻量级的执行流,它可以让程序同时执行多个任务,从而提高程序的效率。线程的启动和关闭是 Python 多线程编程中的核心问题。其中关闭线程是一种常见的需求,因为有时候我们需要手动结束一个线程,例如:线程执行的任务已经完成,或者线程的执行出现了错误,我们希望手动结束它。
Python中的线程关闭方式与启动方式类似,可以使用线程对象的stop()方法或者将一个状态变量设置为False来结束一个线程。
使用 stop() 方法结束线程:
1. 在线程函数中,使用一个无限循环,不断地执行线程任务。
2. 在一个外部线程中,调用要结束的线程对象的 stop() 方法。
3. stop() 方法会使得线程的执行结束,但是一般并不建议使用这种方式来结束线程,因为它会导致程序的不可预测性和安全性。
使用状态变量结束线程:
1. 在线程函数中,使用一个 while 循环,循环条件为状态变量为 True。
2. 在一个外部线程中修改状态变量为 False,从而使得线程终止循环,退出线程。
下面是一个使用状态变量结束线程的示例代码:
```python
import threading
import time
class MyThread(threading.Thread):
def __init__(self, threadID, name):
threading.Thread.__init__(self)
self.threadID = threadID
self.name = name
self.isStop = False
def run(self):
while self.isStop == False:
print("%s is running" % self.name)
time.sleep(1)
print("%s is stopped" % self.name)
def stop(self):
self.isStop = True
if __name__ == "__main__":
t1 = MyThread(1, "Thread-1")
t2 = MyThread(2, "Thread-2")
t1.start()
t2.start()
time.sleep(10)
t1.stop()
t2.stop()
```
以上代码中,MyThread 类继承自 threading.Thread 类,并且重载了 run() 方法,使用一个无限循环来执行线程任务。同时,MyThread 类还定义了一个状态变量 isStop,用于控制线程的退出。在主线程中,先启动了两个 MyThread 类对象 t1 和 t2,然后等待 10 秒后,调用 t1.stop() 和 t2.stop() 方法,将状态变量设置为 False,从而使得线程停止循环,退出线程。
总结来说,在 Python 中,线程的关闭并非一定要用 stop() 方法,因为 stop() 方法并不能保证线程的安全性和可预测性。使用一个状态变量来控制线程退出是一种更好的方式,可以让程序更加可靠和安全。
pyqt5 线程守护
在 PyQt5 中,可以使用 Python 的多线程模块 `threading` 来创建线程,并通过设置线程的 `daemon` 属性来实现线程的守护。
当一个线程被设置为守护线程时,主线程退出时,守护线程会被自动终止,而不管它是否执行完毕。
下面是示例代码,演示了如何在 PyQt5 中创建一个守护线程:
```python
import sys
import time
import threading
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel
class WorkerThread(threading.Thread):
def __init__(self):
super().__init__()
self.daemon = True # 设置线程为守护线程
def run(self):
# 执行耗时操作
time.sleep(5)
print("Worker thread finished")
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
label = QLabel("Main Window", self)
label.setGeometry(100, 100, 200, 30)
worker_thread = WorkerThread()
worker_thread.start()
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在上面的代码中,我们创建了一个继承自 `threading.Thread` 的 `WorkerThread` 类,并在 `__init__` 方法中将 `self.daemon` 设置为 `True`,表示该线程为守护线程。然后在 `run` 方法中执行耗时操作。
在主窗口类 `MainWindow` 的构造函数中,我们创建了一个 `WorkerThread` 实例,并调用 `start` 方法启动守护线程。
需要注意的是,守护线程在执行完毕前,主线程不会退出。所以在上面的例子中,主窗口会一直显示,直到守护线程执行完毕后,才会退出程序。
希望这个例子能帮助到你!如果还有其他问题,请随时问我。
阅读全文
相关推荐
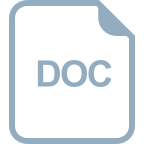
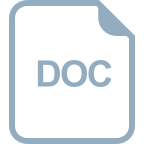
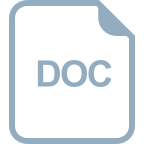
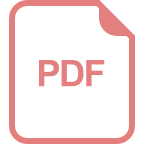
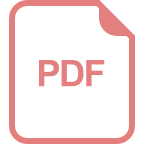
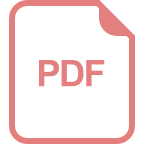
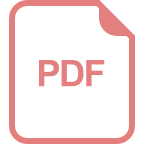
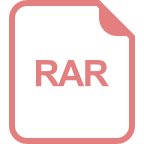
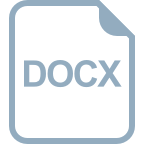
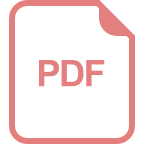
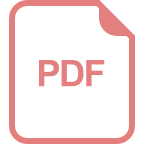
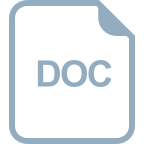
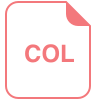
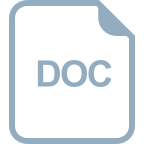
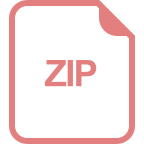
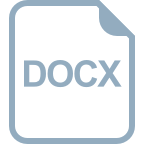