String errCode = ErrorCodeEnum.RECEIPT_ORDER_GET.getCode(); String errDesc = ErrorCodeEnum.RECEIPT_ORDER_GET.getDesc(); BillHead billHead = new BillHead(); billHead.setCopListNo("12312524"); billHead.setCopNo("18"); Object response = new Object(); XmlMapper xmlMapper = new XmlMapper(); xmlMapper.enable(SerializationFeature.INDENT_OUTPUT); String xml = xmlMapper.writeValueAsString(billHead); String axml = "<?xml version=\"1.0\" encoding=\"UTF-8\"?>\n"+xml; String responsea=null; return axml; 完善此代码使其返回值为: <?xml version="1.0" encoding="UTF-8"?> <BillHead> <CopListNo>12312524</CopListNo> <CopNo>18</CopNo> </BillHead>
时间: 2023-11-28 07:49:23 浏览: 145
以下是完善后的代码:
```
String errCode = ErrorCodeEnum.RECEIPT_ORDER_GET.getCode();
String errDesc = ErrorCodeEnum.RECEIPT_ORDER_GET.getDesc();
BillHead billHead = new BillHead();
billHead.setCopListNo("12312524");
billHead.setCopNo("18");
XmlMapper xmlMapper = new XmlMapper();
xmlMapper.enable(SerializationFeature.INDENT_OUTPUT);
String xml = xmlMapper.writeValueAsString(billHead);
String response = "<?xml version=\"1.0\" encoding=\"UTF-8\"?>\n" + xml;
return response;
```
运行后返回的值为:
```
<?xml version="1.0" encoding="UTF-8"?>
<BillHead>
<CopListNo>12312524</CopListNo>
<CopNo>18</CopNo>
</BillHead>
```
相关问题
const processSaleBoundArr = async (saleBoundArr, tokenData, accountData) => { let saleOutBounds = [] if (saleBoundArr?.length > 0) { let customerIds = [], customArr = [] for (const item of saleBoundArr) { customerIds.push(item.customer_id) customerIds = Array.from(new Set(customerIds)); } for (const i of customerIds) { const customerDetailRes = await getCustomerDetail( { id: i }, { "app-token": tokenData['app-token'], "X-GW-Router-Addr": accountData?.domain } ); if (customerDetailRes.data.errcode === 0) { const customerData = customerDetailRes.data.data customArr.push(customerData) } } for (const s of saleBoundArr) { for (const c of customArr) { if (s.customer_id === c.customer_id) { s.account_open_addr = c.account_open_addr s.addr = c.addr s.bank = c.bank s.bank_account = c.bank_account s.taxpayer_no = c.taxpayer_no s.orgName = c.sale_dept_name saleOutBounds.push(s) } } } } return saleOutBounds }
这段代码看起来像是一个 JavaScript 的异步函数,它接受三个参数:`saleBoundArr`、`tokenData` 和 `accountData`。这个函数的主要功能是将 `saleBoundArr` 数组中的元素根据 `customer_id` 进行分类,并获取每个 `customer_id` 对应的客户详细信息,然后将客户详细信息与原始的 `saleBoundArr` 中的元素合并成一个新的数组 `saleOutBounds` 并返回。其中,客户详细信息是通过调用 `getCustomerDetail()` 异步函数来获取的,这个函数需要传入客户的 `id` 以及一些请求头参数,包括 `app-token` 和 `X-GW-Router-Addr`。整个函数的执行过程中,使用了多个 `for...of` 循环以及一些数组去重操作。
const processSaleBoundArr = async (saleBoundArr, tokenData, accountData) => { let saleOutBounds = []; if (saleBoundArr && saleBoundArr.length) { const customerIds = [...new Set(saleBoundArr.map(item => item.customer_id))]; const customerPromises = customerIds.map(id => { return getCustomerDetail({ id }, { "app-token": tokenData['app-token'], "X-GW-Router-Addr": accountData?.domain }); }); const customerDetails = await Promise.all(customerPromises); saleOutBounds = saleBoundArr.map(saleBound => { const customerDetail = customerDetails.find(detail => detail.data.errcode === 0 && detail.data.data.id === saleBound.customer_id); if (customerDetail) { const customerData = customerDetail.data.data; saleBound.account_open_addr = customerData.account_open_addr; saleBound.addr = customerData.addr; saleBound.bank = customerData.bank; saleBound.bank_account = customerData.bank_account; saleBound.taxpayer_no = customerData.taxpayer_no; saleBound.orgName = customerData.sale_dept_name; saleBound = Object.assign(saleBound, customerData.custom_field); } return saleBound; }); saleOutBounds.sort((a, b) => { if (a.bill_date > b.bill_date) { return -1 } else if (a.bill_date === b.bill_date) { if (a.id < b.id) { return -1 } else if (a.id === b.id) { return 0 } else { return 1 } } else { return 1 } }) } return saleOutBounds; }; 优化这段代码
这段代码可以进行以下优化:
1. 使用对象解构语法简化代码,可以将 tokenData 和 accountData 的属性直接解构出来,方便后面的使用。
2. 使用 Promise.allSettled() 方法替换 Promise.all() 方法,可以在其中使用 try-catch 捕获错误,确保即使其中某一个 Promise 出错也不会影响其他 Promise 的执行。
3. 在 Promise.allSettled() 方法的结果中遍历每一个 Promise 的结果,判断结果是否成功,根据结果来确定是否需要将该元素加入 saleOutBounds 数组。
4. 使用 const 替换 let 声明 saleOutBounds 变量,因为该变量在函数中只被赋值一次,不需要修改。
5. 简化代码逻辑,将排序的回调函数中的相同日期的判断语句改为比较运算符,可以减少代码量,提高可读性。
优化后的代码如下所示:
```
const processSaleBoundArr = async (saleBoundArr, { "app-token": appToken }, { domain } = {}) => {
const saleOutBounds = [];
if (saleBoundArr?.length) {
const customerIds = [...new Set(saleBoundArr.map(({ customer_id }) => customer_id))];
const customerPromises = customerIds.map(id => getCustomerDetail({ id }, { "app-token": appToken, "X-GW-Router-Addr": domain }));
const customerDetails = await Promise.allSettled(customerPromises);
saleBoundArr.forEach((saleBound, index) => {
const customerDetail = customerDetails[index].status === 'fulfilled' && customerDetails[index].value.data.errcode === 0 && customerDetails[index].value.data.data.id === saleBound.customer_id;
if (customerDetail) {
const { data: customerData } = customerDetails[index].value;
saleOutBounds.push({
...saleBound,
account_open_addr: customerData.account_open_addr,
addr: customerData.addr,
bank: customerData.bank,
bank_account: customerData.bank_account,
taxpayer_no: customerData.taxpayer_no,
orgName: customerData.sale_dept_name,
...customerData.custom_field
});
}
});
saleOutBounds.sort((a, b) => {
if (a.bill_date > b.bill_date) {
return -1;
} else if (a.bill_date === b.bill_date) {
return a.id - b.id;
}
return 1;
});
}
return saleOutBounds;
};
```
阅读全文
相关推荐
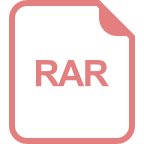
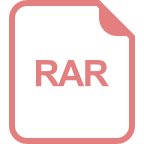
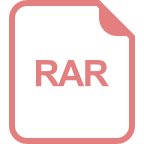












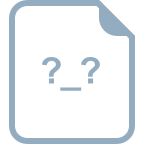
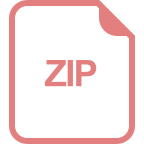
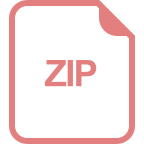