const processSaleBoundArr = async (saleBoundArr, { "app-token": appToken }, { domain } = {}) => { const saleOutBounds = []; if (saleBoundArr?.length) { const customerIds = [...new Set(saleBoundArr.map(({ customer_id }) => customer_id))]; const customerPromises = customerIds.map(id => getCustomerDetail({ id }, { "app-token": appToken, "X-GW-Router-Addr": domain })); const customerDetails = await Promise.allSettled(customerPromises); saleBoundArr.forEach((saleBound, index) => { const customerDetail = customerDetails[index].status === 'fulfilled' && customerDetails[index].value.data.errcode === 0 && customerDetails[index].value.data.data.id === saleBound.customer_id; if (customerDetail) { const { data: customerData } = customerDetails[index].value; saleOutBounds.push({ ...saleBound, account_open_addr: customerData.account_open_addr, addr: customerData.addr, bank: customerData.bank, bank_account: customerData.bank_account, taxpayer_no: customerData.taxpayer_no, orgName: customerData.sale_dept_name, ...customerData.custom_field }); } }); saleOutBounds.sort((a, b) => { if (a.bill_date > b.bill_date) { return -1; } else if (a.bill_date === b.bill_date) { return a.id - b.id; } return 1; }); } return saleOutBounds; }; 捕获异常
时间: 2024-03-13 21:46:44 浏览: 19
在这个函数中,可能会在调用 `getCustomerDetail` 函数时出现错误,也有可能在访问 `customerDetails[index].value` 属性时出现错误。您可以在这两个地方使用 `try-catch` 语句来捕获异常并进行处理。
以下是一个可能的实现方式:
```
const processSaleBoundArr = async (saleBoundArr, { "app-token": appToken }, { domain } = {}) => {
const saleOutBounds = [];
if (saleBoundArr?.length) {
const customerIds = [...new Set(saleBoundArr.map(({ customer_id }) => customer_id))];
const customerPromises = customerIds.map(id => getCustomerDetail({ id }, { "app-token": appToken, "X-GW-Router-Addr": domain }));
try {
const customerDetails = await Promise.allSettled(customerPromises);
saleBoundArr.forEach((saleBound, index) => {
try {
const customerDetail = customerDetails[index].status === 'fulfilled' && customerDetails[index].value.data.errcode === 0 && customerDetails[index].value.data.data.id === saleBound.customer_id;
if (customerDetail) {
const { data: customerData } = customerDetails[index].value;
saleOutBounds.push({
...saleBound,
account_open_addr: customerData.account_open_addr,
addr: customerData.addr,
bank: customerData.bank,
bank_account: customerData.bank_account,
taxpayer_no: customerData.taxpayer_no,
orgName: customerData.sale_dept_name,
...customerData.custom_field
});
}
} catch (error) {
console.error(`Error processing saleBound ${index}:`, error);
}
});
saleOutBounds.sort((a, b) => {
if (a.bill_date > b.bill_date) {
return -1;
} else if (a.bill_date === b.bill_date) {
return a.id - b.id;
}
return 1;
});
} catch (error) {
console.error('Error getting customer details:', error);
}
}
return saleOutBounds;
};
```
在这个实现中,我们在调用 `Promise.allSettled` 和访问 `customerDetails[index].value` 属性时都使用了 `try-catch` 语句来捕获异常,并在控制台输出错误信息。当发生异常时,程序会跳过当前的 saleBound 并继续处理下一个 saleBound。如果您需要在发生异常时中止程序并返回错误,则可以在 `catch` 语句中抛出一个错误。
相关推荐
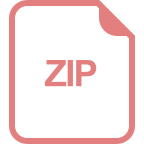
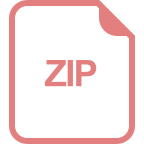
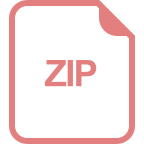















