画布中看到一个黑色圆和一个随机的黑点。等待用户按下F键后,控制台会输出黑点距离圆心的距离和圆心角度,并且扇形会移动到黑点处并变为红色。
时间: 2024-05-08 15:17:50 浏览: 108
这是一个比较复杂的交互式程序,需要使用图形库和键盘输入库来实现。下面是一个可能的实现过程:
1. 导入必要的库:
```python
import math
import random
import pygame
from pygame.locals import *
```
2. 初始化pygame并设置窗口大小:
```python
pygame.init()
WIDTH, HEIGHT = 640, 480
screen = pygame.display.set_mode((WIDTH, HEIGHT))
```
3. 定义圆和黑点的位置和大小:
```python
circle_pos = (WIDTH//2, HEIGHT//2)
circle_radius = 100
black_dot_radius = 10
black_dot_pos = (random.randint(black_dot_radius, WIDTH-black_dot_radius),
random.randint(black_dot_radius, HEIGHT-black_dot_radius))
```
4. 定义计算距离和角度的函数:
```python
def get_distance(pos1, pos2):
x1, y1 = pos1
x2, y2 = pos2
return math.sqrt((x2-x1)**2 + (y2-y1)**2)
def get_angle(pos1, pos2):
x1, y1 = pos1
x2, y2 = pos2
return math.atan2(y2-y1, x2-x1)
```
5. 绘制圆和黑点:
```python
pygame.draw.circle(screen, (0, 0, 0), circle_pos, circle_radius, 1)
pygame.draw.circle(screen, (0, 0, 0), black_dot_pos, black_dot_radius)
```
6. 等待用户按下F键:
```python
while True:
for event in pygame.event.get():
if event.type == KEYDOWN and event.key == K_f:
distance = get_distance(circle_pos, black_dot_pos)
angle = get_angle(circle_pos, black_dot_pos)
print("Distance:", distance)
print("Angle:", angle)
pygame.draw.arc(screen, (255, 0, 0),
pygame.Rect(circle_pos[0]-circle_radius, circle_pos[1]-circle_radius,
2*circle_radius, 2*circle_radius),
angle, angle+math.pi/4, 0)
pygame.display.update()
```
7. 在等待用户按下F键的循环中,如果检测到按键事件,就计算出距离和角度,并输出到控制台。然后绘制一个扇形,表示圆心到黑点的方向,同时将扇形颜色改为红色。最后更新屏幕显示。
完整代码如下:
阅读全文
相关推荐
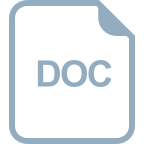
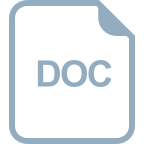
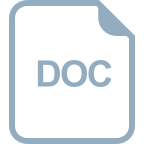

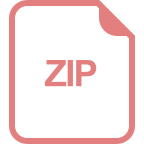
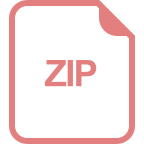
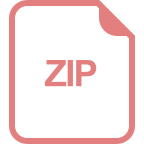
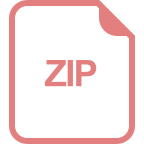
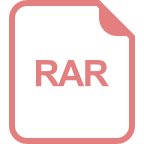
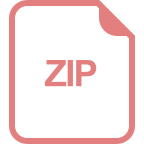
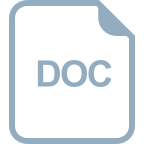
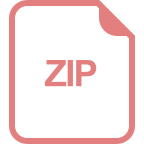
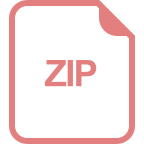
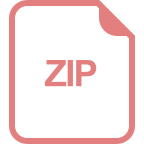
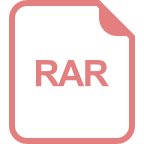
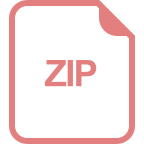
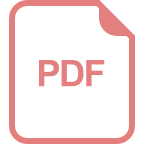

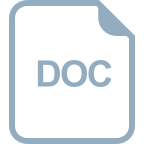