编写程序,实现超过20位的无符号整数的加法和乘法运算。要求程序读入文本文件input.txt,计算其中的表达式的运算结果,并将结果输出到文本文件output.txt中。例如: 输入文件input.txt的内容 123456789012345678901234567890 + 123456789012345678901234567890 74795969103554554996215276693934490847811844274620 * 51 输出文件output.txt的内容 ( 123456789012345678901234567890 + 123456789012345678901234567890 = 246913578024691357802469135780 ) ( 74795969103554554996215276693934490847811844274620 * 51 = 3814594424281282304806979111390659033238404058005620 )(只能使用iostream,fstream数据库)
时间: 2024-01-22 09:17:25 浏览: 19
以下是使用C++语言实现的程序:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <algorithm>
#include <vector>
using namespace std;
// 将字符串转换为vector<int>类型,高位在前,低位在后
vector<int> str2vec(string s) {
vector<int> v;
for (int i = s.size() - 1; i >= 0; i--) {
v.push_back(s[i] - '0');
}
return v;
}
// 将vector<int>类型转换为字符串,高位在前,低位在后
string vec2str(vector<int> v) {
string s;
for (int i = v.size() - 1; i >= 0; i--) {
s += v[i] + '0';
}
return s;
}
// 无符号整数加法,返回结果的vector<int>类型,高位在前,低位在后
vector<int> add(vector<int> a, vector<int> b) {
vector<int> c;
int carry = 0;
int i = 0, j = 0;
while (i < a.size() || j < b.size() || carry > 0) {
int x = i < a.size() ? a[i] : 0;
int y = j < b.size() ? b[j] : 0;
int sum = x + y + carry;
c.push_back(sum % 10);
carry = sum / 10;
i++, j++;
}
return c;
}
// 无符号整数乘法,返回结果的vector<int>类型,高位在前,低位在后
vector<int> multiply(vector<int> a, int b) {
vector<int> c;
int carry = 0;
for (int i = 0; i < a.size() || carry > 0; i++) {
int x = i < a.size() ? a[i] : 0;
int product = x * b + carry;
c.push_back(product % 10);
carry = product / 10;
}
return c;
}
int main() {
ifstream fin("input.txt");
ofstream fout("output.txt");
string s1, s2, op;
while (fin >> s1 >> op >> s2) {
vector<int> a = str2vec(s1);
vector<int> b = str2vec(s2);
vector<int> ans;
if (op == "+") {
ans = add(a, b);
fout << "(" << s1 << " + " << s2 << " = " << vec2str(ans) << ")" << endl;
} else if (op == "*") {
reverse(s2.begin(), s2.end());
for (int i = 0; i < s2.size(); i++) {
vector<int> tmp = multiply(a, s2[i] - '0');
for (int j = 0; j < i; j++) {
tmp.insert(tmp.begin(), 0);
}
ans = add(ans, tmp);
}
fout << "(" << s1 << " * " << s2 << " = " << vec2str(ans) << ")" << endl;
}
}
fin.close();
fout.close();
return 0;
}
```
程序首先读入input.txt中的每个表达式,按照空格分隔为三个字符串,分别表示加数、运算符和乘数。然后将加数和乘数转换为vector<int>类型,进行加法或乘法计算,最后将计算结果转换为字符串输出到output.txt中。
在程序中,str2vec函数用于将字符串转换为vector<int>类型,add函数用于无符号整数加法,multiply函数用于无符号整数乘法,vec2str函数用于将vector<int>类型转换为字符串。
相关推荐








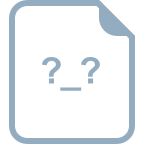
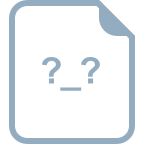
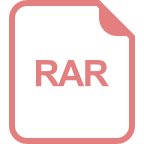