用C语言。从键盘上输入两个字符串(长度不大于20)和整数n(0<n<=20),要求将第二个字符串插在第一个字符串的n位置开始处并输出。 示例如第一个字符串为"you are ok." 第二个字符串为"fine and " 输入的整数为8,则表示将fine插在ok前面(y前面为位置0,o前面的位置为8),输出为you are fine and ok.
时间: 2023-06-07 14:10:53 浏览: 52
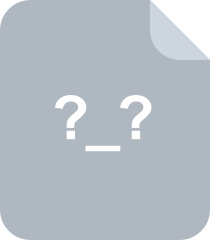
编程实现大整数(128字长)的加、减运算。使得可以实现两个操作数的键盘输入和最终结果的输出。
好的,让我来回答你的问题。
首先我们可以用 scanf 函数从键盘输入两个字符串和一个整数 n,代码如下:
```c
char str1[21], str2[21];
int n;
scanf("%s %s %d", str1, str2, &n);
```
接下来,我们需要将第二个字符串插入第一个字符串的第 n 个位置。我们可以使用 strncpy 函数把第一个字符串从起始位置到 n-1 的位置复制到一个新的字符串中,再把第二个字符串接在该字符串后面,最后再把第一个字符串从第 n 个位置复制到新字符串的末尾。代码如下:
```c
char result[41];
strncpy(result, str1, n);
result[n] = '\0';
strcat(result, str2);
strcat(result, str1 + n);
```
最后,我们将新字符串输出即可:
```c
printf("%s", result);
```
完整代码如下:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str1[21], str2[21];
int n;
scanf("%s %s %d", str1, str2, &n);
char result[41];
strncpy(result, str1, n);
result[n] = '\0';
strcat(result, str2);
strcat(result, str1 + n);
printf("%s", result);
return 0;
}
```
希望能帮到你,如果你还有其他问题可以继续问我。
阅读全文
相关推荐
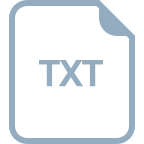




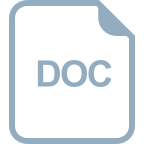
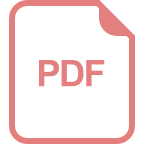
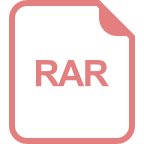
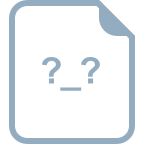
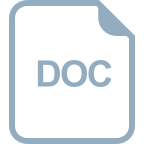
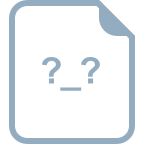
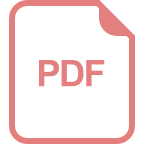
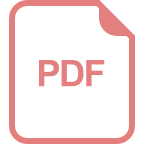
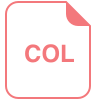

