帮我用Java写一个拳皇游戏
时间: 2023-08-20 19:32:36 浏览: 140
好的,下面是一个简单的拳皇游戏的Java代码,你可以在Java IDE中运行它:
```java
import java.util.*;
import java.io.*;
// 定义拳皇类
class Fighter {
private String name;
private int power;
private int speed;
private int health;
// 构造函数
public Fighter(String name, int power, int speed, int health) {
this.name = name;
this.power = power;
this.speed = speed;
this.health = health;
}
// 获取名称
public String getName() {
return this.name;
}
// 获取力量值
public int getPower() {
return this.power;
}
// 获取速度值
public int getSpeed() {
return this.speed;
}
// 获取生命值
public int getHealth() {
return this.health;
}
// 攻击函数
public void attack(Fighter fighter) {
int damage = this.power - fighter.getSpeed();
if (damage > 0) {
fighter.health -= damage;
System.out.println(this.name + "攻击了" + fighter.getName() + ",造成了" + damage + "点伤害!");
} else {
System.out.println(this.name + "攻击了" + fighter.getName() + ",但是被躲开了!");
}
}
// 判断是否存活
public boolean isAlive() {
return this.health > 0;
}
}
// 定义拳皇游戏类
public class KingOfFighters {
private Fighter player1;
private Fighter player2;
// 构造函数
public KingOfFighters(Fighter player1, Fighter player2) {
this.player1 = player1;
this.player2 = player2;
}
// 游戏开始
public void start() {
System.out.println("游戏开始!");
while (player1.isAlive() && player2.isAlive()) {
player1.attack(player2);
player2.attack(player1);
System.out.println(player1.getName() + "生命值:" + player1.getHealth());
System.out.println(player2.getName() + "生命值:" + player2.getHealth());
}
if (player1.isAlive()) {
System.out.println(player1.getName() + "获胜了!");
} else {
System.out.println(player2.getName() + "获胜了!");
}
}
// 主函数
public static void main(String[] args) {
Fighter player1 = new Fighter("李小龙", 100, 80, 500);
Fighter player2 = new Fighter("布鲁斯李", 90, 90, 500);
KingOfFighters game = new KingOfFighters(player1, player2);
game.start();
}
}
```
这个代码会生成两个拳皇角色对象,然后让它们互相攻击,直到其中一个角色生命值为0,游戏结束并输出获胜者。你可以尝试修改代码中的参数,例如角色的名称、属性值等,来实现不同的效果。
阅读全文
相关推荐
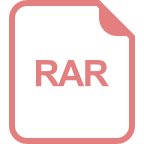
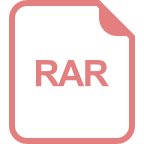

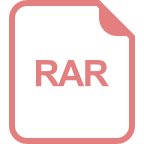
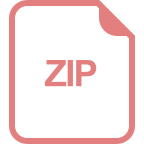
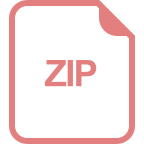
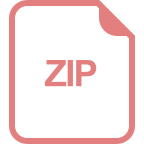
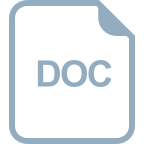
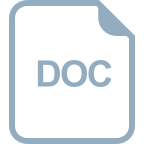
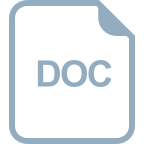
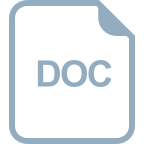

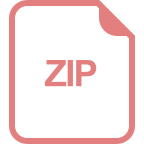
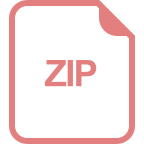