帮我写一段c++代码,使用while(cin.get(ch))读入字符串并储存在字符型数组line中
时间: 2024-04-29 19:24:06 浏览: 83
以下是使用while(cin.get(ch))读入字符串并储存在字符型数组line中的C++代码:
```c++
#include <iostream>
using namespace std;
int main() {
char line[100];
char ch;
int i = 0;
while (cin.get(ch)) {
if (ch == '\n') break;
line[i] = ch;
i++;
}
line[i] = '\0';
cout << "The string you entered is: " << line << endl;
return 0;
}
```
在这段代码中,我们定义了一个字符型数组line,用来存储输入的字符串。我们使用while(cin.get(ch))循环读入字符,如果读入的字符是换行符,就跳出循环。将读入的字符存储在line数组中,同时使用一个计数器i来记录存储的位置。最后,我们在line数组的末尾加上一个空字符'\0',以表示字符串的结束。最后输出读入的字符串。
相关问题
c++ cin.get和cin.getline
cin.get()和cin.getline()都是C++输入函数,它们都是用于从输入流中读取数据,并将其存储在变量中。不同之处在于,它们读取输入数据的方式不同。
cin.get()函数可以读取单个字符或多个字符,并且忽略空格和换行符。它的语法如下:
cin.get(var, size, delimiter);
其中var是用于存储输入数据的字符数组或字符串,size是最大读取字符数,delimiter是可选的分隔符,默认为'\n'。
例如,如果您想要读取一行字符并将其存储在以空格为分隔符的字符数组中,您可以这样写:
char str[100];
cin.get(str, 100, ' ');
cin.getline()函数也可以读取单个字符或多个字符,但它会将换行符('\n')作为结束符,因此它比cin.get()更适用于读取一整行文本。其语法如下:
cin.getline(var, size, delimiter);
其中var是用于存储输入数据的字符数组或字符串,size是最大读取字符数,delimiter是可选的分隔符,默认为'\n'。
例如,如果您想要读取一行字符并将其存储在字符数组中,您可以这样写:
char str[100];
cin.getline(str, 100);
总的来说,cin.get()和cin.getline()都是非常实用的输入函数,在读取字符和行数据时都有各自的优势。您可以根据具体的应用场景选择不同的函数。
C++中的cin.get(),cin.getline(),cin有什么区别分别怎么用
在C++中,`std::cin`是一个标准输入流对象,用于从键盘接收用户输入。`cin.get()`, `cin.getline()`, 和 `cin` 的使用有以下差异:
1. `cin.get()`: 这个函数用于获取单个字符,包括空格和换行符。当你调用`cin.get()`,它会等待用户输入一个字符,然后将这个字符读入并返回。例如:
```cpp
char ch;
cin.get(ch);
```
如果用户按回车键,`ch` 将包含回车符。
2. `cin.getline()`: 这个函数则用于接收一行完整文本(直到遇到换行符为止)。它会读取到一个字符串,并存储在一个字符数组或`std::string`对象中。语法通常是这样的:
```cpp
string inputLine;
getline(cin, inputLine);
```
这里,`inputLine` 就包含了用户按下Enter键前的所有输入。
3. `cin` 直接作为输入流对象使用:你可以直接对它进行操作,如读取整数、浮点数等数据类型。比如,`cin >> num;` 用于读取整数到变量 `num` 中。如果需要换行,可以先调用 `cin.ignore(numeric_limits<streamsize>::max(), '\n');` 来清除缓冲区。
阅读全文
相关推荐
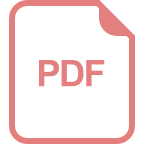
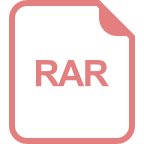
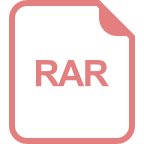
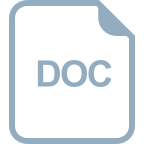
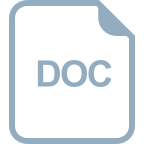

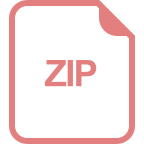
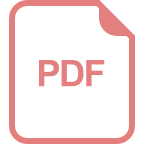
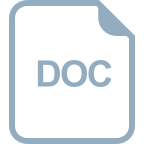
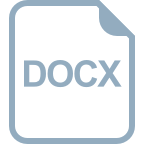
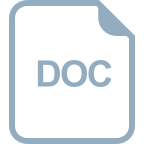
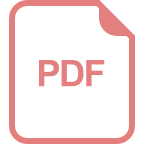