如何在C#中正确地读取和写入网络JSON文件?
时间: 2024-12-30 20:39:18 浏览: 5
在C#中,当你需要从网络上读取或写入JSON文件时,通常会涉及到异步操作,因为网络I/O通常是阻塞的。以下是一个基础示例,展示如何使用HttpClient进行网络请求,并配合Json.NET解析和序列化JSON:
**读取网络JSON文件:**
```csharp
using System.Net.Http;
using Newtonsoft.Json;
public async Task<string> FetchJsonFromWebAsync(string apiUrl)
{
try
{
using HttpClient httpClient = new HttpClient();
HttpResponseMessage response = await httpClient.GetAsync(apiUrl);
response.EnsureSuccessStatusCode(); // 检查HTTP响应状态
using Stream stream = await response.Content.ReadAsStreamAsync();
StreamReader reader = new StreamReader(stream);
return await reader.ReadToEndAsync();
}
catch (HttpRequestException ex)
{
Console.WriteLine($"Error fetching JSON from web: {ex.Message}");
return null;
}
}
// 调用这个方法获取JSON字符串
string jsonString = await FetchJsonFromWebAsync("https://example.com/api/data.json");
dynamic data = JsonConvert.DeserializeObject(jsonString);
```
**写入网络JSON文件:**
```csharp
public async Task UploadJsonToWebAsync(string apiUrl, object jsonContent)
{
try
{
using HttpClient httpClient = new HttpClient();
StringContent content = new StringContent(JsonConvert.SerializeObject(jsonContent), Encoding.UTF8, "application/json");
HttpResponseMessage response = await httpClient.PostAsync(apiUrl, content);
response.EnsureSuccessStatusCode();
Console.WriteLine($"Uploaded JSON to the server at {apiUrl}.");
}
catch (HttpRequestException ex)
{
Console.WriteLine($"Error uploading JSON to web: {ex.Message}");
}
}
// 示例:jsonContent可以是YourClass实例或者其他Json兼容类型
YourClass yourObject = ...;
await UploadJsonToWebAsync("https://example.com/api/upload", yourObject);
```
注意在网络操作中添加适当的错误处理,并可能需要处理网络连接中断、超时等情况。
阅读全文
相关推荐




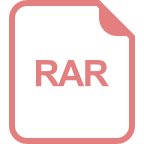












