QXlsx的SheetModel类提供了removeRows()具体代码内容是什么,如果想在sheetmodel里插入含有两个checkbox的代理,并且获得它们的选择状态 草,从而影响另一个单元格设置,代码需要怎么写呢
时间: 2023-09-21 17:05:48 浏览: 235
### 回答1:
1. removeRows()的具体代码如下:
```c++
void SheetModel::removeRows(int row, int count, const QModelIndex &parent)
{
Q_UNUSED(parent);
if (count <= 0 || row < 0 || row >= rowCount()) {
return;
}
int lastrow = row + count - 1;
if (lastrow >= rowCount()) {
lastrow = rowCount() - 1;
}
for (int i = lastrow; i >= row; --i) {
foreach (Cell *cell, m_spreadsheet->cellTable(i)) {
if (cell) {
delete cell;
}
}
m_rows.removeAt(i);
}
emit rowsAboutToBeRemoved(row, lastrow);
emit rowsRemoved(row, lastrow);
emit spreadsheetChanged();
}
```
2. 在SheetModel里插入含有两个checkbox的代理,并且获得它们的选择状态,代码如下:
```c++
// 定义一个自定义的代理类
class CheckBoxDelegate : public QStyledItemDelegate
{
Q_OBJECT
public:
CheckBoxDelegate(QObject *parent = nullptr) : QStyledItemDelegate(parent) {}
QWidget *createEditor(QWidget *parent, const QStyleOptionViewItem &option, const QModelIndex &index) const override
{
QCheckBox *editor = new QCheckBox(parent);
editor->setCheckState(Qt::Unchecked);
return editor;
}
void setEditorData(QWidget *editor, const QModelIndex &index) const override
{
QCheckBox *checkBox = static_cast<QCheckBox *>(editor);
checkBox->setCheckState(index.data(Qt::EditRole).toBool() ? Qt::Checked : Qt::Unchecked);
}
void setModelData(QWidget *editor, QAbstractItemModel *model, const QModelIndex &index) const override
{
QCheckBox *checkBox = static_cast<QCheckBox *>(editor);
model->setData(index, checkBox->isChecked(), Qt::EditRole);
}
void updateEditorGeometry(QWidget *editor, const QStyleOptionViewItem &option, const QModelIndex &index) const override
{
editor->setGeometry(option.rect);
}
};
// 在SheetModel的data()函数中添加代理
QVariant SheetModel::data(const QModelIndex &index, int role) const
{
if (!index.isValid()) {
return QVariant();
}
if (role == Qt::EditRole) {
// 添加代理
if (index.column() == 0 || index.column() == 1) {
CheckBoxDelegate *delegate = new CheckBoxDelegate();
return qVariantFromValue(delegate);
}
}
// 获取checkbox的状态并设置另一个单元格的值
if (role == Qt::DisplayRole) {
if (index.column() == 0 || index.column() == 1) {
QModelIndex otherIndex = this->index(index.row(), 2);
bool isCheckedA = index.siblingAtColumn(0).data(Qt::EditRole).toBool();
bool isCheckedB = index.siblingAtColumn(1).data(Qt::EditRole).toBool();
if (isCheckedA && isCheckedB) {
return "Both A and B are checked";
} else if (isCheckedA) {
return "Only A is checked";
} else if (isCheckedB) {
return "Only B is checked";
} else {
return "None of A and B are checked";
}
}
}
return QAbstractTableModel::data(index, role);
}
```
这样,在SheetModel里对应的单元格就会显示含有两个checkbox的代理,并且可以获取它们的选择状态,从而影响另一个单元格的值。
### 回答2:
QXlsx是一个用于读取、写入和修改Excel文件的库。其中的SheetModel类提供了removeRows()方法用于删除指定行的数据。
removeRows()方法的具体代码内容如下:
```cpp
void SheetModel::removeRows(int row, int row_count, const QModelIndex &parent = QModelIndex())
{
if (row < 0 || row_count <= 0 || row >= m_rows.size())
return;
int end_row = row + row_count - 1;
beginRemoveRows(parent, row, end_row);
m_rows.remove(row, row_count);
endRemoveRows();
}
```
若想在SheetModel中插入一个含有两个checkbox的代理,并且获得它们的选择状态,并根据选择状态影响另一个单元格的设置,可以按以下步骤编写代码。
1. 首先,需要自定义一个代理类,继承自QStyledItemDelegate类。代理类可以重写createEditor()、setEditorData()和setModelData()等方法,以实现自定义编辑器的功能。
2. 在代理类的createEditor()方法中,创建包含两个checkbox的自定义Editor,并将其返回。
3. 在代理类的setEditorData()方法中,从ModelIndex对象中获取checkbox的选择状态,并将状态设置给自定义Editor的两个checkbox。
4. 在代理类的setModelData()方法中,从自定义Editor中获取checkbox的选择状态,并将状态设置给ModelIndex对象。
5. 最后,在SheetModel类中,需要对需要设置代理的单元格进行设置。可以在data()方法中根据条件判断来使某个单元格拥有代理。
这是一个简单的示例,具体的代码实现因具体要求而异。
### 回答3:
QXlsx是一个用于读写Excel文件的开源C++库。SheetModel类是其提供的一个类,用于对Excel表格进行操作。removeRows()方法是SheetModel类的一个成员函数,用于删除指定行。
具体的removeRows()代码实现如下:
```cpp
bool SheetModel::removeRows(int row, int count, const QModelIndex &parent)
{
// 判断行数是否合法
if (row < 0 || row >= rowCount(parent) || count < 1 || row + count >= rowCount(parent))
return false;
// 删除指定行
beginRemoveRows(parent, row, row + count - 1);
m_sheetData->removeRows(row, count);
endRemoveRows();
return true;
}
```
要在SheetModel里插入含有两个checkbox的代理,并且获得它们的选择状态,可以自定义一个继承于QItemDelegate类的代理,并在SheetModel中设置该代理。
具体的代码示例如下:
```cpp
class CheckBoxDelegate : public QItemDelegate
{
public:
QWidget *createEditor(QWidget *parent, const QStyleOptionViewItem &option, const QModelIndex &index) const override
{
QCheckBox *checkBox = new QCheckBox(parent);
return checkBox;
}
void setEditorData(QWidget *editor, const QModelIndex &index) const override
{
QCheckBox *checkBox = qobject_cast<QCheckBox *>(editor);
bool checked = index.data(Qt::EditRole).toBool();
checkBox->setChecked(checked);
}
void setModelData(QWidget *editor, QAbstractItemModel *model, const QModelIndex &index) const override
{
QCheckBox *checkBox = qobject_cast<QCheckBox *>(editor);
bool checked = checkBox->isChecked();
model->setData(index, checked, Qt::EditRole);
}
};
```
然后在SheetModel的构造函数或初始化函数中,为需要用到checkbox的单元格设置代理:
```cpp
CheckBoxDelegate *checkBoxDelegate = new CheckBoxDelegate();
m_sheetView->setItemDelegateForColumn(columnIndex1, checkBoxDelegate);
m_sheetView->setItemDelegateForColumn(columnIndex2, checkBoxDelegate);
```
此时,当用户在表格中勾选或取消勾选checkbox时,可以通过SheetModel获取其选择状态,然后根据需要影响其他单元格的设置。通过获取代理的编辑数据可以实现获取选择状态的功能:
```cpp
bool checked1 = m_sheetModel->data(index1, Qt::EditRole).toBool();
bool checked2 = m_sheetModel->data(index2, Qt::EditRole).toBool();
```
接下来,可以根据需求使用获得的选择状态来影响其他单元格的设置,例如设置另一个单元格的文本值:
```cpp
if (checked1 && checked2) {
m_sheetModel->setData(index3, "选中", Qt::DisplayRole);
} else {
m_sheetModel->setData(index3, "未选中", Qt::DisplayRole);
}
```
以上便是在SheetModel中插入含有两个checkbox的代理,并且获得它们的选择状态,从而影响另一个单元格设置的代码实现。
阅读全文
相关推荐
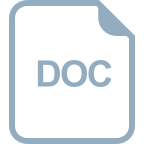

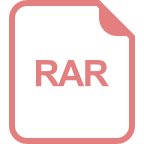
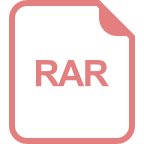















