用Java写一个AES-CMAC加解密代码
时间: 2024-01-28 12:03:04 浏览: 175
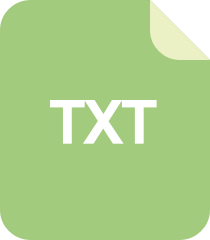
AES加解密的源代码

好的,以下是一个Java实现的AES-CMAC加解密代码示例:
```java
import javax.crypto.*;
import javax.crypto.spec.*;
public class AESCMAC {
private SecretKey key;
public AESCMAC(byte[] keyBytes) throws Exception {
// 创建AES密钥
key = new SecretKeySpec(keyBytes, "AES");
}
public byte[] encrypt(byte[] data) throws Exception {
// 创建加密器
Cipher cipher = Cipher.getInstance("AES/CBC/NoPadding");
cipher.init(Cipher.ENCRYPT_MODE, key);
// 加密数据
byte[] encrypted = cipher.doFinal(data);
// 计算MAC
byte[] mac = calculateCMAC(encrypted);
// 拼接加密数据和MAC
byte[] result = new byte[encrypted.length + mac.length];
System.arraycopy(encrypted, 0, result, 0, encrypted.length);
System.arraycopy(mac, 0, result, encrypted.length, mac.length);
return result;
}
public byte[] decrypt(byte[] data) throws Exception {
// 分离加密数据和MAC
byte[] encrypted = new byte[data.length - 16];
byte[] mac = new byte[16];
System.arraycopy(data, 0, encrypted, 0, encrypted.length);
System.arraycopy(data, encrypted.length, mac, 0, mac.length);
// 计算MAC
byte[] expectedMac = calculateCMAC(encrypted);
// 验证MAC
if (! MessageDigest.isEqual(mac, expectedMac)) {
throw new Exception("Invalid MAC");
}
// 创建解密器
Cipher cipher = Cipher.getInstance("AES/CBC/NoPadding");
cipher.init(Cipher.DECRYPT_MODE, key);
// 解密数据
return cipher.doFinal(encrypted);
}
private byte[] calculateCMAC(byte[] data) throws Exception {
// 创建MAC计算器
Mac mac = Mac.getInstance("AESCMAC");
mac.init(key);
// 计算MAC
byte[] result = mac.doFinal(data);
return result;
}
}
```
使用示例:
```java
// 创建AES-CMAC实例
byte[] keyBytes = new byte[] { 0x01, 0x23, 0x45, 0x67, 0x89, 0xAB, 0xCD, 0xEF, 0xFE, 0xDC, 0xBA, 0x98, 0x76, 0x54, 0x32, 0x10 };
AESCMAC aesCmac = new AESCMAC(keyBytes);
// 加密数据
byte[] data = "Hello, world!".getBytes("UTF-8");
byte[] encrypted = aesCmac.encrypt(data);
System.out.println("Encrypted: " + new String(encrypted, "UTF-8"));
// 解密数据
byte[] decrypted = aesCmac.decrypt(encrypted);
System.out.println("Decrypted: " + new String(decrypted, "UTF-8"));
```
注意:以上代码示例仅供参考,实际使用时需要根据具体需求进行修改和优化。
阅读全文
相关推荐
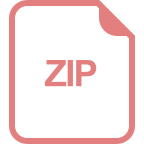
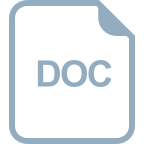
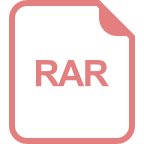
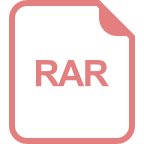
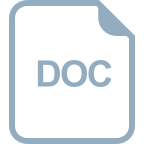

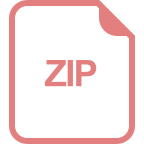
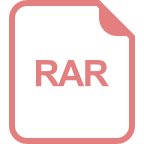
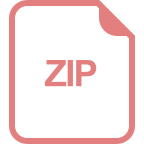
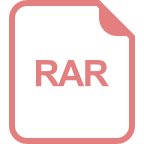
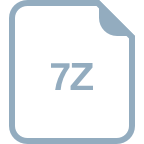
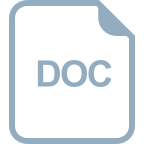
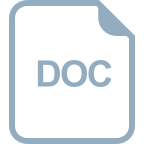
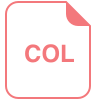
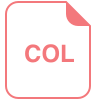
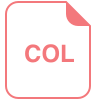