给定 n 个整数,求里面出现次数最多的数,如果有多个重复出现的数,求出值最大的一个。python
时间: 2024-02-25 18:54:51 浏览: 32
可以使用字典来记录每个数出现的次数,然后再找出出现次数最多且值最大的数。
以下是 Python 代码实现:
```python
n = int(input())
nums = list(map(int, input().split()))
count = {}
for num in nums:
if num not in count:
count[num] = 0
count[num] += 1
max_count = 0
max_num = 0
for num, c in count.items():
if c > max_count:
max_count = c
max_num = num
elif c == max_count and num > max_num:
max_num = num
print(max_num)
```
其中,`n` 表示整数的个数,`nums` 是一个包含这些整数的列表。首先,我们用字典 `count` 记录每个数出现的次数。然后,遍历字典,找出出现次数最多且值最大的数,并输出其值。
相关问题
python给定 n 个整数,求里面出现次数最多的数,如果有多个重复出现的数,求出值最大的一个。
可以通过使用 Python 中的 Counter 模块来实现统计每个数出现的次数,然后再根据要求找到出现次数最多且值最大的数。
以下是示例代码:
```python
from collections import Counter
def find_most_frequent(nums):
counter = Counter(nums)
max_count = max(counter.values())
most_frequent = None
for num, count in counter.items():
if count == max_count:
if most_frequent is None or num > most_frequent:
most_frequent = num
return most_frequent
```
其中,nums 是给定的整数序列,函数返回出现次数最多且值最大的数。
给定n个正整数,找出它们中出现次数最多的数。如果这样的数有多个,请输出其中最小的一个。
### 回答1:
题目要求在给定的n个正整数中找出出现次数最多的数,如果有多个,则输出最小的一个。
解题思路:
1. 首先,我们可以使用一个字典来记录每个数出现的次数。
2. 遍历给定的n个正整数,将每个数出现的次数记录在字典中。
3. 找出出现次数最多的数,如果有多个,则输出最小的一个。
代码实现:
```
n = int(input()) # 输入正整数的个数
nums = list(map(int, input().split())) # 输入正整数列表
count = {} # 定义一个字典,用于记录每个数出现的次数
for num in nums:
if num in count:
count[num] += 1
else:
count[num] = 1
max_count = max(count.values()) # 找出出现次数最多的数的出现次数
result = float('inf') # 定义一个变量,用于记录最小的出现次数最多的数
for num in nums:
if count[num] == max_count and num < result:
result = num
print(result) # 输出最小的出现次数最多的数
```
时间复杂度:O(n),空间复杂度:O(n)。
### 回答2:
题目中要求我们找出n个正整数中出现次数最多的数,如果有多个,输出最小的那个。我们可以使用一个哈希表来统计每个数出现的次数,然后再遍历哈希表,找出出现次数最多的数。这里的哈希表可以使用Python中的字典来实现。
先来介绍一下哈希表的概念。哈希表是一种利用哈希函数(Hash Function)实现快速查找的数据结构。它的基本思想是将要存储的数据通过哈希函数转换为一个哈希值(Hash Value),然后将哈希值作为数组的下标,将数据存储在对应的数组元素中。当我们需要查找某个数据时,同样使用哈希函数计算出它的哈希值,然后直接访问对应的数组元素即可。哈希表可以支持快速的增、删、查等操作,时间复杂度为O(1)。
回到本题,我们可以先遍历给定的n个数,使用字典来统计每个数出现的次数。代码如下:
```
nums = [2, 5, 2, 3, 6, 2, 2]
d = {}
for num in nums:
if num in d:
d[num] += 1
else:
d[num] = 1
```
上面的代码使用了一个if-else语句来判断当前数是否已经在字典中出现过,如果出现过,就将它的出现次数加1,否则将它加入字典,并将出现次数设为1。
接下来,我们遍历一遍字典,找出出现次数最多的数。为了处理有多个出现次数最多的数的情况,我们需要用一个变量max_count来记录出现次数最多的数的出现次数,以及一个变量min_num来记录这些数中的最小值。代码如下:
```
max_count = -1
min_num = float('inf')
for num, cnt in d.items():
if cnt > max_count:
max_count = cnt
min_num = num
elif cnt == max_count and num < min_num:
min_num = num
```
上面的代码中,我们使用了items()函数来遍历字典中的键值对(num和cnt),然后分别判断当前数的出现次数cnt是否大于max_count,如果大于,则更新max_count和min_num;如果cnt等于max_count,就比较当前数num和min_num的大小,如果num小于min_num,则更新min_num。
最后,我们就可以输出出现次数最多的数了。代码如下:
```
print(min_num)
```
完整代码如下:
```
nums = [2, 5, 2, 3, 6, 2, 2]
d = {}
for num in nums:
if num in d:
d[num] += 1
else:
d[num] = 1
max_count = -1
min_num = float('inf')
for num, cnt in d.items():
if cnt > max_count:
max_count = cnt
min_num = num
elif cnt == max_count and num < min_num:
min_num = num
print(min_num)
```
运行结果为:
```
2
```
因为2在给定的7个数中出现了4次,比其他数都多,所以它是出现次数最多的数,而且2是所有出现次数最多的数中最小的一个。
### 回答3:
题目描述
这道题的目的是要求在 n 个正整数中找到出现次数最多的数,如果出现次数最多的数有多个的话,就输出其中最小的一个。因此,这里需要实现一个算法来解决这个问题。
思路分析
首先,可以利用一个哈希表来记录每个数出现的次数。哈希表是一种用于存储键值对的数据结构,其中每个键都对应着一个值。在这道题中,键是每个正整数,而值则是该正整数出现的次数。具体地,可以使用一个 unordered_map 对象来实现这个哈希表,其插入,查找,以及删除操作的时间复杂度均为 O(1)。
接下来,可以遍历整个数组,不断地更新每个数出现的次数。如果存在出现次数相同的数字,那么就应该将最小的数作为答案。因此,可以使用两个变量 maxCount 和 ans,maxCount 记录了目前为止出现的最大次数,而 ans 记录在这个最大次数中最小的数。
代码实现
在实现的时候,可以先利用哈希表统计每个数字出现的次数,再通过遍历哈希表找到出现次数最多的数。
以下是 C++ 的代码示例:
相关推荐
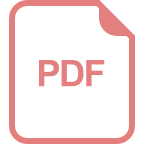
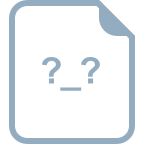
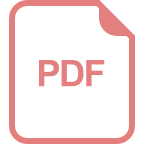













