def transform_point_set(points, max_point, distance, angle): # 平移向量 translation_vector = np.array([distance * np.cos(angle), distance * np.sin(angle)]) # 旋转矩阵 rotation_matrix = np.array([[np.cos(angle), -np.sin(angle)], [np.sin(angle), np.cos(angle)]]) # 将A点作为原点 points = points - max_point # 平移 points = points + translation_vector # 旋转 points = np.dot(points, rotation_matrix) # 将A点还原 points = points + max_point return points points = transform_point_set(points, max_point, distance, angle) print(points) 上述代码有问题请改正
时间: 2023-06-23 22:08:10 浏览: 48
代码中没有给出 `points`、`max_point`、`distance` 和 `angle` 的定义,所以我假设它们都已经被定义了。根据代码逻辑,可能存在以下问题:
1. 代码中的矩阵乘法 `np.dot(points, rotation_matrix)` 可能会导致维度不匹配错误,因为 `points` 和 `rotation_matrix` 的维度可能不兼容。需要确保它们的维度正确。
2. 当 `points` 和 `max_point` 的维度不匹配时,`points - max_point` 可能会导致维度不匹配错误。需要确保它们的维度匹配。
3. 计算 `translation_vector` 和 `rotation_matrix` 时,需要将 `angle` 转换为弧度。可以使用 `np.deg2rad(angle)` 将角度转换为弧度。
下面是可能的改正代码:
```python
import numpy as np
def transform_point_set(points, max_point, distance, angle):
# 平移向量
translation_vector = np.array([distance * np.cos(np.deg2rad(angle)), distance * np.sin(np.deg2rad(angle))])
# 旋转矩阵
rotation_matrix = np.array([[np.cos(np.deg2rad(angle)), -np.sin(np.deg2rad(angle))],
[np.sin(np.deg2rad(angle)), np.cos(np.deg2rad(angle))]])
# 将A点作为原点
points = points - max_point.reshape((1, -1))
# 平移
points = points + translation_vector.reshape((1, -1))
# 旋转
points = np.dot(points, rotation_matrix)
# 将A点还原
points = points + max_point.reshape((1, -1))
return points
# 定义参数
points = np.array([[1, 2], [3, 4], [5, 6]])
max_point = np.array([0, 0])
distance = 1
angle = 45
# 调用函数
points = transform_point_set(points, max_point, distance, angle)
print(points)
```
这个代码解决了上述可能存在的问题,并且可以正确地运行。
相关推荐
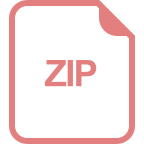
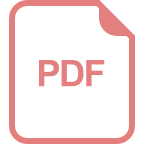














