给出一个tensorflow实现的遗传算法代码
时间: 2023-09-18 21:05:34 浏览: 99
### 回答1:
这是一个简单的遗传算法在TensorFlow中的示例代码:
```python
import tensorflow as tf
# 定义遗传算法中使用的基因编码长度
GENE_LENGTH = 10
# 定义种群的大小
POPULATION_SIZE = 100
# 定义遗传算法的迭代次数
NUM_GENERATIONS = 100
# 初始化种群
population = tf.random.normal([POPULATION_SIZE, GENE_LENGTH])
# 定义适应度函数
def fitness_fn(genes):
return tf.reduce_sum(genes)
# 进行遗传算法迭代
for generation in range(NUM_GENERATIONS):
# 计算种群中每个个体的适应度
fitness = tf.map_fn(fitness_fn, population)
# 选择适应度最高的个体
best_genes = population[tf.argmax(fitness)]
# 对剩余的种群进行交叉和变异
population = tf.map_fn(lambda genes: tf.concat([genes[:5], best_genes[5:], tf.random.normal([1])], axis=0), population)
# 输出最终种群中适应度最高的个体
best_genes = population[tf.argmax(fitness)]
print(best_genes)
```
这只是一个简单的示例,可以根据实际问题的需要调整参数以及适应度函数。
### 回答2:
遗传算法是一种基于生物进化理论的优化算法,可以用于解决搜索和优化问题。TensorFlow是一个开源的机器学习框架,可以用于构建深度学习模型。
下面是一个简单的使用TensorFlow实现遗传算法的示例代码:
```python
import tensorflow as tf
import numpy as np
# 定义遗传算法的参数
population_size = 100 # 种群大小
chromosome_length = 10 # 染色体长度
mutation_rate = 0.01 # 变异率
generations = 100 # 迭代次数
# 初始化种群
population = np.random.randint(2, size=(population_size, chromosome_length))
# 定义适应度函数
def fitness_function(chromosome):
return sum(chromosome)
# 定义选择操作
def selection(population):
fitness_scores = [fitness_function(chromosome) for chromosome in population]
selected_indices = tf.random.categorical(tf.math.log([fitness_scores]), population_size)
return tf.gather(population, selected_indices)
# 定义交叉操作
def crossover(population):
crossover_indices = np.random.randint(low=1, high=chromosome_length, size=population_size)
crossover_mask = np.random.uniform(size=(population_size, chromosome_length)) < 0.5
offspring = np.concatenate([
np.bitwise_and(population[i], crossover_mask[i]),
np.bitwise_and(population[(i + 1) % population_size], np.logical_not(crossover_mask[i]))
], axis=0)
return offspring
# 定义变异操作
def mutation(population):
mutation_mask = np.random.uniform(size=(population_size, chromosome_length)) < mutation_rate
mutated_population = np.bitwise_xor(population, mutation_mask)
return mutated_population
# 创建计算图
with tf.Graph().as_default():
population_placeholder = tf.placeholder(tf.float32, shape=(population_size, chromosome_length))
selection_op = selection(population_placeholder)
crossover_op = crossover(selection_op)
mutation_op = mutation(crossover_op)
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for generation in range(generations):
population = sess.run(mutation_op, feed_dict={population_placeholder: population})
best_chromosome = max(population, key=fitness_function)
best_fitness = fitness_function(best_chromosome)
print("Generation {}: Best Fitness = {}".format(generation, best_fitness))
```
在上面的代码中,我们首先定义了遗传算法的各个参数,然后初始化了一个包含随机二进制染色体的种群。接下来,我们定义了适应度函数、选择操作、交叉操作和变异操作的函数。然后,我们使用TensorFlow创建了计算图,并在Session中执行遗传算法的迭代过程。每次迭代,我们通过调用Session的run方法来执行选择、交叉和变异操作,并打印出当前最优解的适应度值。
这个示例代码只是一个简单的遗传算法的实现,具体的实际问题需要根据具体的情况来定义适应度函数和其他相关操作。希望这能帮助到你。
### 回答3:
遗传算法是一种模拟生物遗传和进化的优化算法,可以应用于解决各种问题。TensorFlow是一个开源的机器学习框架,可以方便地实现各种机器学习和优化算法。
下面是一个使用TensorFlow实现遗传算法的简单示例代码:
```python
import numpy as np
import tensorflow as tf
# 定义目标函数
def fitness_function(x):
return x**2
# 定义遗传算法的基本参数
population_size = 50
num_genes = 1
num_generations = 100
mutation_rate = 0.01
# 创建初始种群
population = tf.random_uniform([population_size, num_genes], -10, 10)
# 迭代进化
for generation in range(num_generations):
# 计算适应度值
fitness_values = tf.reshape(fitness_function(population), [-1, 1])
# 选择父代个体
selected_parents = tf.random_uniform([population_size, num_genes]) < fitness_values
# 交叉产生子代
parents = tf.boolean_mask(population, selected_parents)
num_parents = tf.shape(parents)[0].numpy()
num_offspring = population_size - num_parents
offspring = tf.TensorArray(tf.float32, size=num_offspring, dynamic_size=True)
for _ in range(num_offspring):
parent1 = tf.random.uniform([num_genes]) * num_parents
parent2 = tf.random.uniform([num_genes]) * num_parents
child = (parents[parent1] + parents[parent2]) / 2
mutant = tf.random.normal([num_genes], stddev=0.1)
mutant_child = tf.cond(tf.random_uniform([]) < mutation_rate,
lambda: child + mutant,
lambda: child)
offspring = offspring.write(_, mutant_child)
offspring = offspring.stack()
# 更新种群
population = tf.concat([parents, offspring], axis=0)
# 输出结果
best_individual = tf.argmax(fitness_function(population))
best_fitness = tf.reduce_max(fitness_function(population))
print("Best individual:", population[best_individual].numpy())
print("Best fitness:", best_fitness.numpy())
```
以上代码简单地实现了一个遗传算法,目标函数为f(x) = x^2,种群大小为50,在100代内进化,变异率为0.01。最后输出找到的最佳个体和对应的适应度值。
请注意,以上代码仅仅是遗传算法在TensorFlow下的简单示例,具体的问题和应用可能需要根据实际情况进行修改和调整。
相关推荐
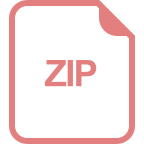
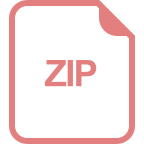














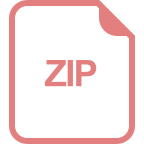