使用TensorFlow实现遗传算法解决TSP问题的简单示例代码
时间: 2023-02-09 16:16:27 浏览: 238
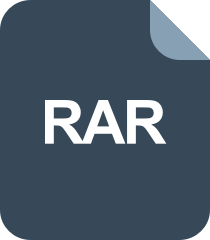
一个用遗传算法解决TSP的程序
以下是使用 TensorFlow 实现遗传算法解决 TSP 问题的示例代码:
```
import tensorflow as tf
import numpy as np
# 初始化城市坐标
cities = tf.constant([[1, 2], [3, 4], [5, 6], [7, 8], [9, 10]], dtype=tf.float32)
# 随机初始化旅行顺序
def init_population():
return tf.random.shuffle(tf.range(tf.shape(cities)[0]))
# 计算路径长度
def path_length(path):
indices = tf.stack([path[:-1], path[1:]], axis=-1)
distances = tf.gather_nd(cities, indices)
return tf.reduce_sum(tf.norm(distances, axis=-1))
# 遗传算法的交叉操作
def crossover(parents):
# 随机选取交叉点
cross_point = tf.random.uniform([], 0, tf.shape(parents)[-1], dtype=tf.int32)
# 将父代串连接在一起
parents = tf.concat(parents, axis=-1)
# 将父代串按交叉点分割
children = tf.split(parents, [cross_point, tf.shape(parents)[-1] - cross_point], axis=-1)
# 将两个子代的基因重新组合
children = tf.concat(children[::-1], axis=-1)
# 消除重复基因
_, children = tf.unique(children)
return children
# 遗传算法的变异操作
def mutation(children):
# 随机选取两个基因交换位置
indices = tf.random.shuffle(tf.range(tf.shape(children)[-1]))[:2]
children = tf.tensor_scatter_nd_update(children, tf.expand_dims(indices, axis=-1), tf.reverse(tf.gather(children, indices), axis=[-1]))
return children
# 遗传算法的选择操作
def selection(population, scores):
# 计算累积概率
prob = tf.cumsum(scores) / tf.reduce_sum(scores)
# 随机选取下一代种
阅读全文
相关推荐

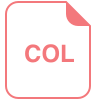
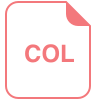
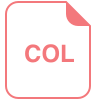
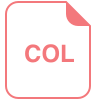
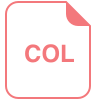
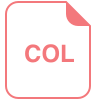
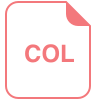
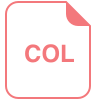
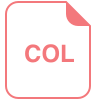