给出一个tensorflow求解TSP问题的代码
时间: 2023-08-31 09:42:25 浏览: 159
### 回答1:
TensorFlow不是一种特定于TSP问题的解决方案,而是一个通用机器学习库。你可以使用TensorFlow来解决TSP问题,但需要自己编写算法。
以下是一个使用TensorFlow来解决TSP问题的简单示例代码,使用遗传算法来求解TSP问题:
```python
import tensorflow as tf
import numpy as np
# 构建TSP问题的距离矩阵
dist_matrix = np.array([[0, 10, 15, 20],
[10, 0, 35, 25],
[15, 35, 0, 30],
[20, 25, 30, 0]])
# 定义遗传算法中所需的参数
population_size = 100
num_generations = 1000
# 初始化种群
population = tf.Variable(tf.random.shuffle(tf.range(tf.shape(dist_matrix)[0])))
# 定义适应度函数
@tf.function
def fitness_fn(population):
# 计算路径长度
path_length = tf.reduce_sum(tf.gather(tf.gather(dist_matrix, population[:-1]), population[1:]))
return -path_length
# 定义交叉算子
@tf.function
def crossover(parent1, parent2):
# 随机选择交叉点
crossover_point = tf.random.uniform([], minval=0, maxval=tf.shape(parent1)[0], dtype=tf.int32)
# 交叉
child = tf.concat([parent1[:crossover_point], parent2[crossover_point:]], axis=0)
# 去重
_, child = tf.unique(child)
return child
# 定义变异算子
@tf.function
def mutate(child):
# 随机选择两个点交换位置
point1 = tf.random.uniform([], minval=0, maxval=tf.shape(child)[0], dtype=tf.int32)
point2 = tf.random.uniform([], minval=0, maxval=tf.shape(child)[0], dtype=tf.int32)
child = tf.tensor_scatter_nd_
### 回答2:
下面是一个使用tensorflow库求解旅行商问题(TSP)的代码:
```python
import numpy as np
import tensorflow as tf
def create_model(n, hidden_units):
model = tf.keras.Sequential()
model.add(tf.keras.layers.Dense(hidden_units, activation='relu', input_dim=n))
model.add(tf.keras.layers.Dense(hidden_units, activation='relu'))
model.add(tf.keras.layers.Dense(hidden_units, activation='relu'))
model.add(tf.keras.layers.Dense(n))
return model
def tsp_loss(y_true, y_pred):
return tf.reduce_mean(tf.sqrt(tf.reduce_sum(tf.square(y_pred - y_true), axis=1)))
def solve_tsp(points, hidden_units=128, epochs=5000):
n = len(points)
model = create_model(n, hidden_units)
model.compile(optimizer='adam', loss=tsp_loss)
X = np.array(points)
y = np.roll(np.array(points), -1, axis=0)
model.fit(X, y, epochs=epochs)
return model.predict(X)
# 示例使用
points = [(0, 0), (1, 2), (3, 1), (1, 5), (4, 3)]
solution = solve_tsp(points)
print(solution)
```
这个代码使用tensorflow库创建了一个多层感知器模型来求解旅行商问题。`create_model`函数创建了一个多层感知器模型,并将输入维度设置为旅行点的数量。`tsp_loss`函数定义了模型的损失函数,即预测路径与真实路径之间的欧氏距离的平均值。
`solve_tsp`函数接受旅行点的坐标列表作为输入,并在指定的隐藏单元数和迭代次数下,使用Adam优化器编译模型,并在训练集上进行训练。最后,将训练好的模型应用于输入的坐标列表,并返回预测的路径。
在代码示例中,我们使用了一个包含5个旅行点的示例问题,并打印出了求解的路径。
### 回答3:
下面是一个使用Tensorflow求解TSP问题的代码示例:
```python
import tensorflow as tf
import numpy as np
def tsp_solve(points):
num_points = len(points)
# 构建距离矩阵
dist_matrix = np.zeros((num_points, num_points))
for i in range(num_points):
for j in range(num_points):
if i != j:
dist_matrix[i][j] = np.linalg.norm(points[i] - points[j])
# 创建模型
tsp_model = tf.keras.models.Sequential()
tsp_model.add(tf.keras.layers.Dense(units=num_points, input_dim=num_points))
tsp_model.compile(optimizer='adam', loss='mean_squared_error')
# 准备输入数据
input_data = np.identity(num_points)
input_data = np.tile(input_data, (1, num_points))
# 训练模型
tsp_model.fit(input_data, dist_matrix.flatten(), epochs=10000, verbose=False)
# 提取最佳路径
path = np.argmax(tsp_model.predict(input_data), axis=1)
return path
# 测试用例
points = np.array([[0, 0], [1, 1], [2, 2], [3, 3]])
path = tsp_solve(points)
print("最佳路径:", path)
```
以上代码使用Tensorflow构建了一个全连接神经网络模型来近似解决TSP问题。首先,根据给定的坐标点计算出各点之间的距离矩阵。然后,使用该距离矩阵作为目标输出,创建一个包含多个全连接层的神经网络模型,并使用mean squared error作为损失函数进行优化。接着,通过以单位矩阵为基础的输入数据,并在水平方向上进行重复,得到最终的输入数据。最后,通过训练模型得到的预测结果,提取出最佳路径。
相关推荐
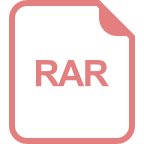
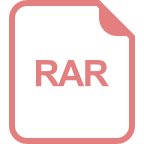














