写一个多线程java下载文件
时间: 2023-11-27 09:39:56 浏览: 38
好的,可以的。以下是Java多线程下载文件的示例代码:
public class DownloadManager {
public static void main(String[] args) {
String url = "https://sample.com/sample.pdf"; // 下载链接
String saveDir = "C:/Downloads/"; // 保存目录
int threadCount = 4; // 线程数
int bufferSize = 1024; // 缓冲区大小
DownloadTask downloadTask = new DownloadTask(url, saveDir, threadCount, bufferSize);
downloadTask.download();
}
}
class DownloadTask {
private String url;
private String saveDir;
private int threadCount;
private int bufferSize;
public DownloadTask(String url, String saveDir, int threadCount, int bufferSize) {
this.url = url;
this.saveDir = saveDir;
this.threadCount = threadCount;
this.bufferSize = bufferSize;
}
public void download() {
try {
URL downloadUrl = new URL(url);
HttpURLConnection conn = (HttpURLConnection) downloadUrl.openConnection();
conn.setRequestMethod("GET");
conn.setConnectTimeout(5000);
int length = conn.getContentLength();
System.out.println("文件长度:" + length);
File file = new File(saveDir + conn.getHeaderField("Content-Disposition").split("filename=")[1]);
RandomAccessFile raf = new RandomAccessFile(file, "rw");
raf.setLength(length);
raf.close();
int blockSize = length % threadCount == 0 ? length / threadCount : length / threadCount + 1;
for (int threadId = 0; threadId < threadCount; threadId++) {
int startIndex = blockSize * threadId;
int endIndex = Math.min(startIndex + blockSize - 1, length - 1);
new DownloadThread(url, file, startIndex, endIndex, bufferSize).start();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
class DownloadThread extends Thread {
private String url;
private File file;
private int startIndex;
private int endIndex;
private int bufferSize;
public DownloadThread(String url, File file, int startIndex, int endIndex, int bufferSize) {
this.url = url;
this.file = file;
this.startIndex = startIndex;
this.endIndex = endIndex;
this.bufferSize = bufferSize;
}
@Override
public void run() {
try {
URL downloadUrl = new URL(url);
HttpURLConnection conn = (HttpURLConnection) downloadUrl.openConnection();
conn.setRequestMethod("GET");
conn.setConnectTimeout(5000);
conn.setRequestProperty("Range", "bytes=" + startIndex + "-" + endIndex);
InputStream is = conn.getInputStream();
RandomAccessFile raf = new RandomAccessFile(file, "rw");
raf.seek(startIndex);
byte[] buffer = new byte[bufferSize];
int len;
while ((len = is.read(buffer)) != -1) {
raf.write(buffer, 0, len);
}
raf.close();
is.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
相关推荐
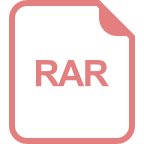














