Implementation and efficiency analysis of quicksort algorithm(快速排序算法实现及效率分析)Another good example of recursion is quicksort, a sorting algorithmdeveloped by C.A.R. Hoare in 1962. Given an array, one element is chosen andthe others partitioned in two subsets - those less than the partition element andthose greater than or equal to it. The same process is then applied recursively tothe two subsets. When a subset has fewer than two elements, it doesn't need anysorting; this stops the recursion.要求:随机产生N(N<=100000)个整数并保存到文本文件中,然后从这个文件中读入这N个整数,并把排序结果保存到另一个文本文件中,并显示排序所用的时间。
时间: 2024-02-14 10:09:25 浏览: 144
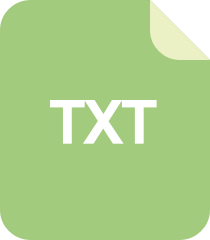
快速排序的一个算法(一个例子)
好的,您的问题是如何实现快速排序算法并进行效率分析,同时使用随机生成的整数进行排序并将结果保存到文件中。我可以帮您解决这个问题。
首先介绍一下快速排序算法的基本思想:首先在数组中选择一个元素作为基准值,然后把数组中小于基准值的元素放在它的左边,大于基准值的元素放在它的右边。然后对左右两个子集递归地进行同样的操作,直到子集中只有一个元素或者为空。快速排序的时间复杂度为O(nlogn),是一种比较高效的排序算法。
下面是快速排序算法的实现代码(使用C++语言):
```cpp
#include <iostream>
#include <fstream>
#include <ctime>
#include <cstdlib>
using namespace std;
void quicksort(int arr[], int left, int right) {
if (left >= right) return; // 递归终止条件
int i = left, j = right, pivot = arr[left];
while (i < j) {
while (i < j && arr[j] >= pivot) j--;
if (i < j) arr[i++] = arr[j];
while (i < j && arr[i] < pivot) i++;
if (i < j) arr[j--] = arr[i];
}
arr[i] = pivot;
quicksort(arr, left, i - 1);
quicksort(arr, i + 1, right);
}
int main() {
const int N = 100000; // 元素个数
int arr[N];
srand(time(NULL)); // 用系统时间初始化随机数种子
ofstream fout("input.txt"); // 打开输出文件流
for (int i = 0; i < N; i++) {
arr[i] = rand(); // 生成随机数
fout << arr[i] << endl; // 写入文件
}
fout.close(); // 关闭输出文件流
clock_t start, finish;
start = clock(); // 记录开始时间
quicksort(arr, 0, N - 1); // 快速排序
finish = clock(); // 记录结束时间
cout << "Time used: " << (double)(finish - start) / CLOCKS_PER_SEC << "s" << endl;
ofstream fout2("output.txt"); // 打开输出文件流
for (int i = 0; i < N; i++) {
fout2 << arr[i] << endl; // 写入文件
}
fout2.close(); // 关闭输出文件流
return 0;
}
```
在这个代码中,我们首先生成N个随机数并保存到文件“input.txt”中,然后读入这些随机数并进行快速排序操作,最后将排序结果保存到文件“output.txt”中。同时,我们使用clock()函数来记录排序所用的时间,单位为秒。
需要注意的是,当N的值较大时,排序时间可能会比较长,甚至可能超过几分钟。因此,我们可以考虑使用更为高效的排序算法,如归并排序、堆排序等。同时,我们还可以使用多线程等技术来加速排序过程。
希望这个回答能够帮助您解决问题!
阅读全文
相关推荐
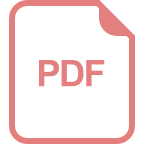
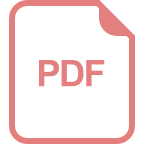
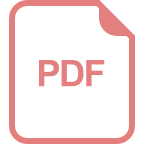
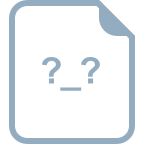
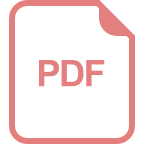
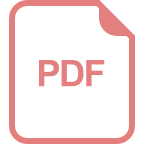
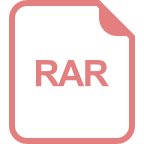
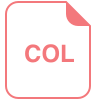
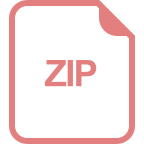
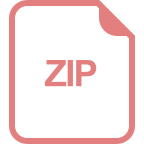
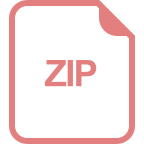