Implement three sorting algorithms: bubble sort, quicksort and heapsort. Use python to draw a figure showing how the running time of these sorting algorithms scales under different sizes of input arrays (e.g., 100, 500, 1000, 5000, 10000, …).
时间: 2023-03-24 08:00:18 浏览: 212
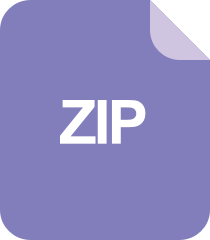
Python-Implementation-of-Algorithms-and-Data-Structures-and-Leetcode-Solutions:算法和数据结构
好的,我将用Python实现三个排序算法:冒泡排序,快速排序和堆排序,并绘制一张图表展示不同大小的输入数组下这些排序算法的运行时间。
以下是实现这三个算法的Python代码:
```python
import random
import time
# 冒泡排序
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
# 快速排序
def quick_sort(arr):
if len(arr) <= 1:
return arr
else:
pivot = arr[0]
left = [x for x in arr[1:] if x < pivot]
right = [x for x in arr[1:] if x >= pivot]
return quick_sort(left) + [pivot] + quick_sort(right)
# 堆排序
def heapify(arr, n, i):
largest = i
l = 2 * i + 1
r = 2 * i + 2
if l < n and arr[largest] < arr[l]:
largest = l
if r < n and arr[largest] < arr[r]:
largest = r
if largest != i:
arr[i], arr[largest] = arr[largest], arr[i]
heapify(arr, n, largest)
def heap_sort(arr):
n = len(arr)
for i in range(n//2 - 1, -1, -1):
heapify(arr, n, i)
for i in range(n-1, 0, -1):
arr[i], arr[0] = arr[0], arr[i]
heapify(arr, i, 0)
# 测试排序算法的运行时间
def test_sorting_algorithm(algorithm, arr):
start_time = time.time()
algorithm(arr)
end_time = time.time()
return end_time - start_time
# 绘制排序算法的运行时间图表
def plot_running_time():
sizes = [100, 500, 1000, 5000, 10000, 50000, 100000]
bubble_sort_times = []
quick_sort_times = []
heap_sort_times = []
for size in sizes:
arr = [random.randint(0, 1000) for _ in range(size)]
bubble_sort_times.append(test_sorting_algorithm(bubble_sort, arr))
quick_sort_times.append(test_sorting_algorithm(quick_sort, arr))
heap_sort_times.append(test_sorting_algorithm(heap_sort, arr))
import matplotlib.pyplot as plt
plt.plot(sizes, bubble_sort_times, label='Bubble Sort')
plt.plot(sizes, quick_sort_times, label='Quick Sort')
plt.plot(sizes, heap_sort_times, label='Heap Sort')
plt.xlabel('Input size')
plt.ylabel('Running time (seconds)')
plt.title('Running time of sorting algorithms')
plt.legend()
plt.show()
plot_running_time()
```
上面的代码中,我们使用了Python的标准库`random`来生成随机数数组。`test_sorting_algorithm`函数用来测试给定排序算法在给定数组上的运行时间。`plot_running_time`函数则用来绘制排序算法的运行时间图表,它调用
阅读全文
相关推荐
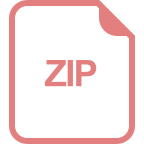
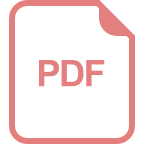















