Please briefly describe the idea of the algorithm and implement it through programming(use java)
时间: 2024-10-09 08:15:05 浏览: 18
The provided pseudo-code snippet suggests a method to compute the reflexive transitive closure (`R*`) of a binary relation `R` over a finite set `A`. The key concept here is adding all pairs `(ai, ai)` (i.e., making the relation reflexive by ensuring every element relates to itself) to `R*`, but this alone only ensures reflexivity. To fully achieve both reflexivity and transitivity, we need to ensure that if there's a path from `ai` to `aj`, then `(ai, aj)` should be in `R*`.
Here's a basic implementation using Java:
```java
import java.util.*;
public class ReflexiveTransitiveClosure {
public static Set<Pair> computeReflexiveTransitiveClosure(Set<Pair> R, int n) {
// Initialize R* with the reflexive part.
Set<Pair> RStar = new HashSet<>();
for(int i = 1; i <= n; i++) {
RStar.add(new Pair(i, i));
}
// Add transitive elements to R* based on existing relations in R.
boolean changes;
do {
changes = false;
Set<Pair> temp = new HashSet<>(RStar);
for(Pair p : R) {
for(Pair q : R) {
if(p.y == q.x && !temp.contains(new Pair(p.x, q.y))) {
temp.add(new Pair(p.x, q.y)); // Add new transitive pair.
changes = true;
}
}
}
RStar = temp;
} while(changes);
return RStar;
}
public static void main(String[] args) {
// Example usage:
int n = 3; // Size of set A
Set<Pair> R = new HashSet<>(); // Initial binary relation R
// Assume R contains these pairs initially
R.add(new Pair(1, 2));
R.add(new Pair(2, 3));
Set<Pair> RStar = computeReflexiveTransitiveClosure(R, n);
System.out.println("Computed Reflexive Transitive Closure: " + RStar);
}
static class Pair {
int x, y;
Pair(int x, int y) {
this.x = x;
this.y = y;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof Pair)) return false;
Pair pair = (Pair) o;
return x == pair.x && y == pair.y;
}
@Override
public int hashCode() {
return Objects.hash(x, y);
}
@Override
public String toString() {
return "("+x+", "+y+")";
}
}
}
```
In this code, `computeReflexiveTransitiveClosure` function first adds all reflexive pairs `(ai, ai)` to `R*`. Then it enters a loop where it checks for transitive closures until no more such pairs are found to add. This process continues until `R*` stabilizes, indicating all necessary transitive pairs have been added, thereby completing the reflexive transitive closure computation.
阅读全文
相关推荐
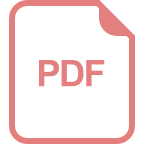
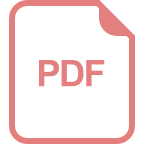

















