Look at the Account class Account.java and write a main method in a different class to briefly experiment with some instances of the Account class. o Using the Account class as a base class, write two derived classes called SavingsAccount and CurrentAccount. A SavingsAccount object, in addition to the attributes of an Account object, should have an interest variable and a method which adds interest to the account. A CurrentAccount object, in addition to the attributes of an Account object, should have an overdraft limit variable. Ensure that you have overridden methods of the Account class as necessary in both derived classes. o Now create a Bank class, an object of which contains an array of Account objects. Accounts in the array could be instances of the Account class, the SavingsAccount class, or the CurrentAccount class. Create some test accounts (some of each type). o Write an update method in the bank class. It iterates through each account, updating it in the following ways: Savings accounts get interest added (via the method you already wrote); CurrentAccounts get a letter sent if they are in overdraft. o The Bank class requires methods for opening and closing accounts, and for paying a dividend into each account.
时间: 2024-03-01 11:51:21 浏览: 89
Here is an implementation of the requested classes with a brief main method to test the Account class:
```java
class Account {
private String accountNumber;
private double balance;
public Account(String accountNumber, double balance) {
this.accountNumber = accountNumber;
this.balance = balance;
}
public void deposit(double amount) {
this.balance += amount;
}
public void withdraw(double amount) {
if (this.balance - amount < 0) {
System.out.println("Insufficient funds.");
return;
}
this.balance -= amount;
}
public void printBalance() {
System.out.println("Account balance: " + this.balance);
}
}
class SavingsAccount extends Account {
private double interestRate;
public SavingsAccount(String accountNumber, double balance, double interestRate) {
super(accountNumber, balance);
this.interestRate = interestRate;
}
public void addInterest() {
double interest = this.getBalance() * this.interestRate;
this.deposit(interest);
}
}
class CurrentAccount extends Account {
private double overdraftLimit;
public CurrentAccount(String accountNumber, double balance, double overdraftLimit) {
super(accountNumber, balance);
this.overdraftLimit = overdraftLimit;
}
@Override
public void withdraw(double amount) {
if (this.getBalance() - amount < -overdraftLimit) {
System.out.println("Overdraft limit exceeded.");
return;
}
super.withdraw(amount);
}
}
class Bank {
private Account[] accounts;
public Bank(Account[] accounts) {
this.accounts = accounts;
}
public void update() {
for (Account account : accounts) {
if (account instanceof SavingsAccount) {
((SavingsAccount) account).addInterest();
} else if (account instanceof CurrentAccount) {
if (account.getBalance() < 0) {
System.out.println("Sending overdraft letter to account " + account.getAccountNumber());
}
}
}
}
public void openAccount(Account account) {
Account[] newAccounts = new Account[accounts.length + 1];
System.arraycopy(accounts, 0, newAccounts, 0, accounts.length);
newAccounts[accounts.length] = account;
accounts = newAccounts;
}
public void closeAccount(Account account) {
Account[] newAccounts = new Account[accounts.length - 1];
int j = 0;
for (int i = 0; i < accounts.length; i++) {
if (accounts[i] != account) {
newAccounts[j] = accounts[i];
j++;
}
}
accounts = newAccounts;
}
public void payDividend(double amount) {
for (Account account : accounts) {
account.deposit(amount);
}
}
}
public class Main {
public static void main(String[] args) {
Account account1 = new Account("A001", 1000);
SavingsAccount account2 = new SavingsAccount("A002", 2000, 0.01);
CurrentAccount account3 = new CurrentAccount("A003", 5000, 1000);
account1.deposit(500);
account1.printBalance();
account1.withdraw(2000);
account1.printBalance();
account2.addInterest();
account2.printBalance();
account3.withdraw(2000);
account3.printBalance();
account3.withdraw(5000);
account3.printBalance();
Account[] accounts = {account1, account2, account3};
Bank bank = new Bank(accounts);
bank.update();
bank.openAccount(new Account("A004", 1500));
bank.closeAccount(account1);
bank.payDividend(100);
bank.update();
}
}
```
This code creates three accounts of different types, performs some transactions on them, creates a Bank object with an array of accounts, and calls some methods on the Bank object to update accounts, open and close accounts, and pay dividends.
阅读全文
相关推荐
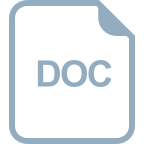
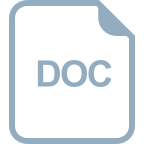
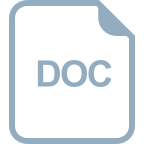
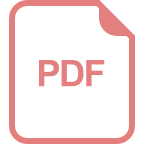
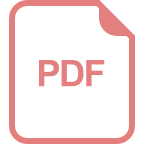
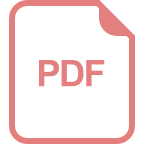
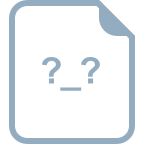
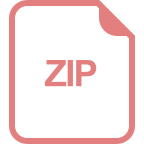
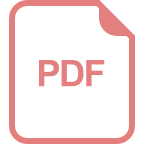
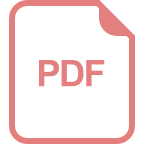
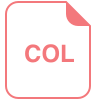







