linux usb转串口编程
时间: 2023-10-23 11:29:41 浏览: 39
在Linux上进行USB转串口编程需要使用libusb库和串口通信库,比如libserialport库。
首先需要安装libusb和libserialport库,可以使用以下命令进行安装:
```
sudo apt-get install libusb-dev libserialport-dev
```
然后可以使用libusb库来获取USB设备的信息,比如设备ID和端口号。接下来,需要打开串口设备并进行读写操作。可以使用libserialport库来打开串口设备,设置串口参数,读写数据等。下面是一个简单的示例代码:
```
#include <stdio.h>
#include <string.h>
#include <errno.h>
#include <unistd.h>
#include <libusb-1.0/libusb.h>
#include <libserialport.h>
#define VENDOR_ID 0x1234
#define PRODUCT_ID 0x5678
int main(int argc, char **argv) {
int ret;
struct libusb_device_handle *dev_handle;
struct sp_port *serial_port;
// 初始化libusb库
ret = libusb_init(NULL);
if (ret < 0) {
fprintf(stderr, "libusb_init error: %s\n", libusb_error_name(ret));
return -1;
}
// 查找USB设备
dev_handle = libusb_open_device_with_vid_pid(NULL, VENDOR_ID, PRODUCT_ID);
if (dev_handle == NULL) {
fprintf(stderr, "libusb_open_device_with_vid_pid error\n");
libusb_exit(NULL);
return -1;
}
// 获取USB设备端口号
int port = libusb_get_port_number(libusb_get_device(dev_handle));
printf("Device port number: %d\n", port);
// 打开串口设备
serial_port = sp_new();
if (serial_port == NULL) {
fprintf(stderr, "sp_new error\n");
libusb_close(dev_handle);
libusb_exit(NULL);
return -1;
}
ret = sp_open(serial_port, "/dev/ttyUSB0", SP_MODE_READ_WRITE);
if (ret < 0) {
fprintf(stderr, "sp_open error: %s\n", sp_last_error_message());
sp_free(serial_port);
libusb_close(dev_handle);
libusb_exit(NULL);
return -1;
}
// 设置串口参数
sp_set_baudrate(serial_port, 115200);
sp_set_bits(serial_port, 8);
sp_set_parity(serial_port, SP_PARITY_NONE);
sp_set_stopbits(serial_port, 1);
// 读写数据
char buf[256];
size_t nbytes;
nbytes = sp_blocking_read(serial_port, buf, sizeof(buf), 1000);
if (nbytes > 0) {
printf("Read %zd bytes from serial port: %.*s\n", nbytes, (int)nbytes, buf);
}
nbytes = snprintf(buf, sizeof(buf), "Hello, world!");
ret = sp_blocking_write(serial_port, buf, nbytes, 1000);
if (ret < 0) {
fprintf(stderr, "sp_blocking_write error: %s\n", sp_last_error_message());
}
// 关闭串口设备和USB设备,释放资源
sp_close(serial_port);
sp_free(serial_port);
libusb_close(dev_handle);
libusb_exit(NULL);
return 0;
}
```
在代码中,VENDOR_ID和PRODUCT_ID分别为USB设备的厂商ID和产品ID,可以通过lsusb命令查看。/dev/ttyUSB0为串口设备的路径,可以根据实际情况进行修改。在读写数据时,可以使用sp_blocking_read和sp_blocking_write函数进行阻塞式读写操作。
注意:在运行程序前需要将当前用户添加到dialout用户组中,命令为:
```
sudo adduser <username> dialout
```
其中<username>为当前用户名。这样才能访问串口设备。
相关推荐
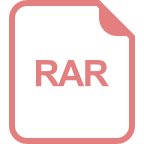













