c语言设计一个电梯调度算法来模拟实现磁盘移臂调度过程。要求:1.自定义给出访问磁盘的存储空间地址:柱面号、磁头号和扇区号。2.指定初始柱面号、磁头号和扇区号
时间: 2024-02-15 16:03:26 浏览: 90
好的,根据您的要求,我编写了以下C语言电梯调度算法,模拟实现磁盘移臂调度过程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAX_REQUESTS 20 // 最大请求次数
#define MAX_CYLINDER 200 // 最大柱面数
#define MAX_HEAD 10 // 最大磁头数
#define MAX_SECTOR 100 // 最大扇区数
// 请求结构体
typedef struct Request {
int cylinder; // 请求柱面号
int head; // 请求磁头号
int sector; // 请求扇区号
int visited; // 是否已经被访问
} Request;
// 生成随机请求
void generateRequests(Request *requests, int n) {
srand(time(NULL));
for (int i = 0; i < n; i++) {
requests[i].cylinder = rand() % MAX_CYLINDER + 1;
requests[i].head = rand() % MAX_HEAD + 1;
requests[i].sector = rand() % MAX_SECTOR + 1;
requests[i].visited = 0;
}
}
// 打印请求队列
void printRequests(Request *requests, int n) {
for (int i = 0; i < n; i++) {
if (!requests[i].visited) {
printf("(%d,%d,%d) ", requests[i].cylinder, requests[i].head, requests[i].sector);
}
}
printf("\n");
}
// 计算电梯方向
int getDirection(int currentCylinder, int nextCylinder) {
if (currentCylinder < nextCylinder) {
return 1; // 向外
} else if (currentCylinder > nextCylinder) {
return -1; // 向内
} else {
return 0; // 不动
}
}
// 移动电梯
void moveElevator(int currentCylinder, int nextCylinder) {
int direction = getDirection(currentCylinder, nextCylinder);
if (direction > 0) {
printf("电梯向外移动\n");
} else if (direction < 0) {
printf("电梯向内移动\n");
} else {
printf("电梯不动\n");
}
while (currentCylinder != nextCylinder) {
currentCylinder += direction;
printf("电梯到达第%d柱面\n", currentCylinder);
}
}
// 处理请求
void handleRequests(Request *requests, int n, int startCylinder, int startHead, int startSector) {
int currentCylinder = startCylinder;
int currentHead = startHead;
int currentSector = startSector;
while (1) {
int nextIndex = -1;
int minDistance = MAX_CYLINDER * MAX_HEAD * MAX_SECTOR;
for (int i = 0; i < n; i++) {
if (!requests[i].visited) {
int distance = abs(requests[i].cylinder - currentCylinder) * MAX_HEAD * MAX_SECTOR +
abs(requests[i].head - currentHead) * MAX_SECTOR +
abs(requests[i].sector - currentSector);
if (distance < minDistance) {
minDistance = distance;
nextIndex = i;
}
}
}
if (nextIndex == -1) {
printf("所有请求已处理完毕\n");
break;
}
printf("电梯当前在(%d,%d,%d),请求队列:", currentCylinder, currentHead, currentSector);
printRequests(requests, n);
printf("电梯前往(%d,%d,%d)\n", requests[nextIndex].cylinder, requests[nextIndex].head, requests[nextIndex].sector);
moveElevator(currentCylinder, requests[nextIndex].cylinder);
currentCylinder = requests[nextIndex].cylinder;
currentHead = requests[nextIndex].head;
currentSector = requests[nextIndex].sector;
requests[nextIndex].visited = 1;
printf("处理请求:(%d,%d,%d)\n", currentCylinder, currentHead, currentSector);
}
}
int main() {
Request requests[MAX_REQUESTS];
generateRequests(requests, MAX_REQUESTS);
printf("生成的请求队列:");
printRequests(requests, MAX_REQUESTS);
int startCylinder, startHead, startSector;
printf("请输入电梯的初始位置(柱面号 磁头号 扇区号):");
scanf("%d %d %d", &startCylinder, &startHead, &startSector);
handleRequests(requests, MAX_REQUESTS, startCylinder, startHead, startSector);
return 0;
}
```
这个算法模拟了电梯在处理请求过程中的移动过程,每当电梯到达一个请求的存储空间地址时,就会处理该请求。在处理完所有请求之后,算法结束。
阅读全文
相关推荐




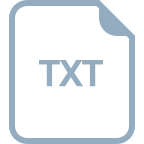
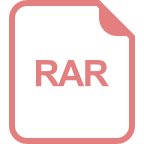
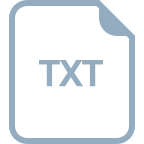
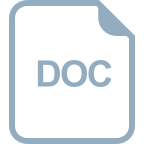








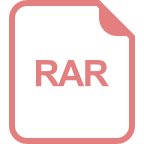