unity 读取pdf
时间: 2023-06-30 08:17:53 浏览: 124
Unity 自身并没有提供直接读取 PDF 文件的 API,但可以利用第三方库实现该功能。
一种可行的方案是使用 `PdfiumViewer`,它是一个基于 `Pdfium` 的 .NET PDF 渲染器,可以在 Unity 中使用。以下是使用 `PdfiumViewer` 在 Unity 中读取 PDF 文件的示例代码:
1. 首先,需要将 `PdfiumViewer` 库添加到 Unity 项目中。可以将该库添加为本地包或使用 NuGet 安装。具体步骤如下:
- 将 `PdfiumViewer.dll` 和 `PdfiumViewer.xml` 文件复制到 Unity 项目的 Assets 文件夹中;
- 在 Unity 项目中创建一个名为 `Plugins` 的文件夹,并将 `PdfiumViewer.dll` 文件移动到该文件夹中;
- 在 Visual Studio 中使用 NuGet 安装 `PdfiumViewer`。
2. 创建一个名为 `PdfViewer` 的 C# 脚本,并添加以下代码:
```c#
using System.IO;
using System.Linq;
using System.Runtime.InteropServices;
using System.Threading.Tasks;
using PdfiumViewer;
public class PdfViewer : MonoBehaviour
{
public string filePath = "MyPdf.pdf";
public int pageIndex = 0;
public int resolution = 72;
private PdfDocument document;
async void Start()
{
await LoadPdfAsync(filePath);
RenderPdfPage(pageIndex, resolution);
}
async Task LoadPdfAsync(string path)
{
if (document != null)
document.Dispose();
using (var stream = File.OpenRead(path))
document = await Task.Run(() => PdfDocument.Load(stream));
}
void RenderPdfPage(int index, int dpi)
{
if (document == null)
return;
using (var page = document.Pages[index])
using (var bitmap = new System.Drawing.Bitmap((int)(page.Width * dpi / 72.0f), (int)(page.Height * dpi / 72.0f)))
using (var graphics = System.Drawing.Graphics.FromImage(bitmap))
{
graphics.Clear(System.Drawing.Color.White);
page.Render(graphics, dpi, dpi, 0, 0, bitmap.Width, bitmap.Height, false);
var imageData = BitmapToByteArray(bitmap);
// TODO: 处理图像数据,例如显示在 UI 上
}
}
byte[] BitmapToByteArray(System.Drawing.Bitmap bitmap)
{
var data = bitmap.LockBits(new System.Drawing.Rectangle(0, 0, bitmap.Width, bitmap.Height), System.Drawing.Imaging.ImageLockMode.ReadOnly, bitmap.PixelFormat);
var buffer = new byte[data.Stride * data.Height];
Marshal.Copy(data.Scan0, buffer, 0, buffer.Length);
bitmap.UnlockBits(data);
return buffer;
}
void OnDestroy()
{
if (document != null)
document.Dispose();
}
}
```
3. 将 `PdfViewer` 脚本挂载到场景中的 GameObject 上,并设置 `filePath`、`pageIndex` 和 `resolution` 变量。其中,`filePath` 是 PDF 文件的路径,`pageIndex` 是要渲染的页面索引,`resolution` 是渲染分辨率。
4. 运行 Unity 项目即可看到 PDF 页面的渲染结果。可以根据需要对图像数据进行处理,例如显示在 UI 上。
需要注意的是,`PdfiumViewer` 库是一个较大的库,使用时需要注意项目体积和性能。另外,由于该库是基于 .NET 的,因此在 Unity Web Player 或 Unity WebGL 等平台上可能会遇到兼容性问题。
希望这个解决方案能够帮助到你。
相关推荐
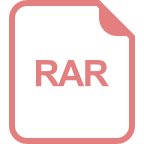
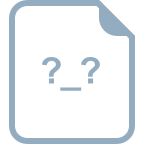
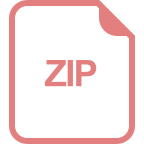














