编写程序输入n个整数链式存放并输出最大值
时间: 2023-05-31 13:17:47 浏览: 322
### 回答1:
首先,需要定义一个链表节点的结构体,包含一个整数值和指向下一个节点的指针。代码如下:
```
struct Node {
int value;
Node* next;
};
```
然后,需要输入n个整数并将它们存储在链表中。可以使用一个循环来实现,每次输入一个整数,创建一个新的节点并将其添加到链表的末尾。代码如下:
```
int n;
cin >> n;
Node* head = nullptr;
Node* tail = nullptr;
for (int i = ; i < n; i++) {
int value;
cin >> value;
Node* node = new Node{value, nullptr};
if (head == nullptr) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
```
最后,需要遍历链表并找到最大值。可以使用一个循环来遍历链表,每次比较当前节点的值和最大值,如果当前节点的值更大,则更新最大值。代码如下:
```
int max_value = head->value;
Node* current = head->next;
while (current != nullptr) {
if (current->value > max_value) {
max_value = current->value;
}
current = current->next;
}
cout << "最大值为:" << max_value << endl;
```
完整代码如下:
```
#include <iostream>
using namespace std;
struct Node {
int value;
Node* next;
};
int main() {
int n;
cin >> n;
Node* head = nullptr;
Node* tail = nullptr;
for (int i = ; i < n; i++) {
int value;
cin >> value;
Node* node = new Node{value, nullptr};
if (head == nullptr) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
int max_value = head->value;
Node* current = head->next;
while (current != nullptr) {
if (current->value > max_value) {
max_value = current->value;
}
current = current->next;
}
cout << "最大值为:" << max_value << endl;
return ;
}
```
### 回答2:
题目要求我们编写程序输入n个整数链式存放并输出最大值。这是一道非常经典的算法题,我们可以通过编写代码来解决这个问题。
首先,我们需要定义一个链表节点的结构体,包含一个整数数据域和指向下一个节点的指针域。代码如下:
```
struct ListNode {
int val;
ListNode* next;
};
```
接下来,我们可以通过循环读取数据来构造链表。我们可以定义一个头节点作为链表的起点,然后一次将输入的整数插入链表中。代码如下:
```
int n;
cin >> n;
ListNode* head = new ListNode();
ListNode* cur = head;
for (int i = 0; i < n; i++) {
int val;
cin >> val;
cur->next = new ListNode();
cur->next->val = val;
cur = cur->next;
}
```
接下来,我们需要遍历整个链表,找到其中的最大值。我们可以使用一个变量来保存当前已经遍历过的节点中的最大值,并与当前节点的值比较,如果当前节点的值比最大值大,则更新最大值。代码如下:
```
int maxVal = INT_MIN;
cur = head->next;
while (cur) {
if (cur->val > maxVal) {
maxVal = cur->val;
}
cur = cur->next;
}
```
最后,我们输出找到的最大值即可。完整的代码如下:
```
#include <iostream>
#include <climits>
using namespace std;
struct ListNode {
int val;
ListNode* next;
};
int main() {
int n;
cin >> n;
ListNode* head = new ListNode();
ListNode* cur = head;
for (int i = 0; i < n; i++) {
int val;
cin >> val;
cur->next = new ListNode();
cur->next->val = val;
cur = cur->next;
}
int maxVal = INT_MIN;
cur = head->next;
while (cur) {
if (cur->val > maxVal) {
maxVal = cur->val;
}
cur = cur->next;
}
cout << maxVal << endl;
return 0;
}
```
综上所述,编写程序输入n个整数链式存放并输出最大值的问题可以通过链表的遍历来解决,代码实现比较简单,但需要注意一些细节。
### 回答3:
要实现输入 n 个整数链式存放的功能,可以采用链表数据结构进行存储。链表是由多个节点组成的,每个节点都有两个部分:一个存储数据的部分和一个指向下一个节点的指针。在这个问题中,我们可以设计一个名为 Node 的结构体,表示每个节点。结构体 Node 中应至少包含数据部分、指针部分和访问它们的方法。以下是一个示例 Node 结构体:
```
typedef struct node {
int data;
struct node* next;
} Node;
```
接下来,需要编写程序输入 n 个整数,并将它们链式存放到链表中。如果输入的整数数目是可得知的,则可以使用 for 循环进行输入。如果不知道数目,则可以使用 while 循环连续读取输入,直到用户输入结束标记。在读取每个整数后仍需动态创建新节点并添加到链表中,这可以通过以下代码实现:
```
Node* head = NULL;
Node* tail = NULL;
int n, value;
scanf("%d", &n);
for(int i = 0; i < n; i++) {
scanf("%d", &value);
Node* new_node = (Node*)malloc(sizeof(Node));
new_node->data = value;
new_node->next = NULL;
if(tail == NULL) {
head = new_node;
tail = new_node;
} else {
tail->next = new_node;
tail = new_node;
}
}
```
以上代码中,head 和 tail 分别指向链表的头部和尾部,其初始值均为 NULL。当用户输入第一个整数时,创建一个新节点并将其赋值为头部和尾部;余下的整数创建新节点并追加到链表中。这样,就完成了链表的创建。
最后,我们需要在链表中找到最大值并输出。遍历链表可实现该操作,并且对于 n 个整数的输入,时间复杂度可以达到 O(n)。以下是查找最大值并输出的代码实现:
```
int maxValue = head->data;
Node* current = head->next;
while(current != NULL) {
if(current->data > maxValue) {
maxValue = current->data;
}
current = current->next;
}
printf("The maximum value is %d", maxValue);
```
以上代码中,我们将 head 中存储的值设定为当前最大值,并从链表的第二个节点开始遍历所有节点。如果当前节点的值大于当前最大值,则将 current 中存储的值设定为新的最大值。最后,输出最大值。
综上所述,通过使用链表数据结构,我们可以实现输入 n 个整数链式存放并输出最大值的功能。需要注意的是,在使用动态内存分配时一定要小心,要避免出现内存泄漏和悬挂指针等问题。
相关推荐
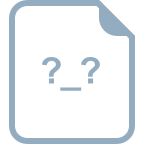













