#include <iostream> using namespace std; class Animal { //int age; /********** Begin **********/ private: int age; /********** End **********/ }; class Dog : Animal { public: void SetAge(int n) {age = n;} void ShowAge() {cout<<age;} }; int main() { int n; Dog d; cin>>n; d.SetAge(n); d.ShowAge(); return 0; }补全
时间: 2023-07-05 17:31:33 浏览: 79
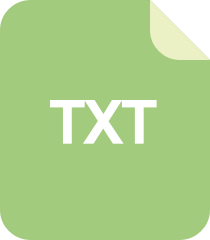
使用include <iostream>时一定要加using namespace std
这段代码看起来是一个简单的面向对象编程的例子,实现了一个 Dog 类,其中包含了一个私有成员变量 age,以及两个公有成员函数 SetAge 和 ShowAge,用于设置和显示 age 的值。另外,Dog 类继承自 Animal 类,但是在这个例子中 Animal 类并没有定义任何成员变量和成员函数。
如果要补全这段代码,可以考虑为 Animal 类和 Dog 类添加一些新的成员变量和成员函数,使得它们更加完整和功能丰富。例如,可以为 Animal 类添加一个名为 name 的成员变量,用于表示动物的名称;为 Dog 类添加一个名为 breed 的成员变量,用于表示狗的品种。
此外,还可以添加一些其他的成员函数,例如 Animal 类中添加一个名为 Eat 的成员函数,用于模拟动物进食的过程;Dog 类中添加一个名为 Bark 的成员函数,用于模拟狗叫的声音。
最终的代码可能如下所示:
```
#include <iostream>
using namespace std;
class Animal {
private:
int age;
string name;
public:
Animal(int a, string n) : age(a), name(n) {}
int GetAge() { return age; }
string GetName() { return name; }
void Eat() { cout << name << " is eating." << endl; }
};
class Dog : Animal {
private:
string breed;
public:
Dog(int a, string n, string b) : Animal(a, n), breed(b) {}
string GetBreed() { return breed; }
void Bark() { cout << GetName() << " is barking." << endl; }
};
int main() {
int age;
string name, breed;
cin >> age >> name >> breed;
Dog d(age, name, breed);
d.Eat();
d.Bark();
cout << "Age: " << d.GetAge() << endl;
cout << "Name: " << d.GetName() << endl;
cout << "Breed: " << d.GetBreed() << endl;
return 0;
}
```
在主函数中,我们首先从标准输入中读入一个整数 age、一个字符串 name 和一个字符串 breed,然后通过调用 Dog 类的构造函数创建一个 Dog 对象 d。接着,我们调用 d 对象的 Eat 和 Bark 成员函数,模拟狗的进食和叫声。最后,我们分别调用 d 对象的 GetAge、GetName 和 GetBreed 成员函数,将狗的年龄、名称和品种输出到标准输出中。
阅读全文
相关推荐
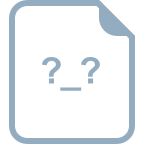
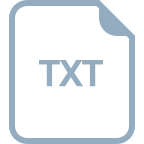





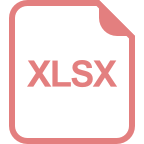
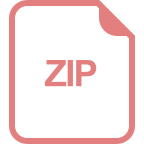
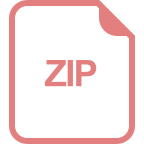
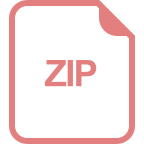
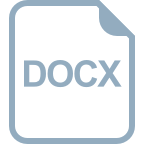
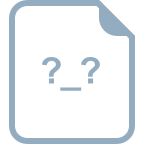
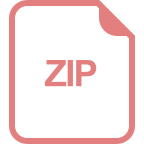
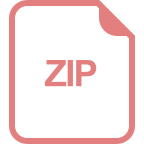
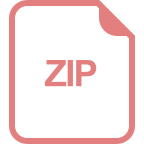